Since its first appearance in Xojo 2020r1, one of the most demanded features for PDFDocument
has been the ability to add Table Of Content entries. With Xojo 2021r3 that’s now possible, and pretty straightforward! Continue reading and I will show you how.
When we think about Table Of Content (TOC) entries, they can clearly be seen as a tree hierarchy where each branch denotes a deeper level hanging from a parent node.
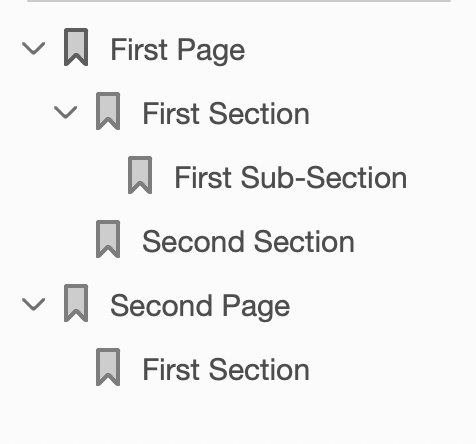
In the above image, see how the “First Page” and “Second Page” entries in the TOC are at a root level for the first page and second pages in the document, respectively; while both “First Section” and “Second Section” are one level deeper, hanging from the root entry, and “First Sub-Section” is a third level deeper, hanging in this case from the “First Section” entry on the “First Page”.
How can we create this kind of TOC hierarchy in a PDFDocument? Using the PDFTOCEntry
class in combination with the PDFDocument.AddTOCEntry
method.
The PDFTOCEntry
Constructor takes a total of four parameters:
- PageNumber As Integer. This is the page number of the
PDFDocument
instance that will be associated with the TOC entry. PDF pages start at 1. - Title As String. The text that will be displayed as the title for the TOC entry in the PDF viewer.
- X As Integer. The X coordinate where the PDF viewer will jump in the associated page when the user clicks the TOC entry in the PDF viewer.
- Y As Integer. The Y coordinate where the PDF viewer will jump in the associated page when the user clicks the TOC entry in the PDF Viewer.
We will return a bit later to see how the “jump” to the X and Y coordinates behave in the PDF Viewer.
So, in order to create our first root TOC entry for the first page in a PDFDocument instance, all we need to do is:
Var te As New PDFTOCEntry(1,"First Page",0,40)
Now, the “First Section” entry means that we want to add a Bookmark (or TOC entry) to the PDFDocument that, once the user clicks on it, will jump to a specific point (X/Y coordinates) in the same page; so we can create a new instance for it:
Var te1 As New PDFTOCEntry(1,"First Section",100,100)
And the same thing for the first sub-section that will hang from the “First Section” entry:
Var te1a As New PDFTOCEntry(1,"First Sub-Section", 0, 100)
So far, we have three PDFTOCEntry
instances, but we still haven’t set any hierarchy among these. For that, use the PDFTOCEntry.AddEntry
method. The following line of code will add the te1a
instance as a sub-node on the te1
instance (that is, “First Sub-Section” as a node of “First Section”):
te1.AddEntry te1a
And now we can add the te1
instance as a sub-node of the te
instance (that is “First Section” will be a sub-node of “First Page”):
te.AddEntry te1
Thus, in order to add a new section named “Second Section” also as a sub-node for the “First Page” root node, we only need to type the following lines of code:
Var te2 As New PDFTOCEntry(1,"Second Section", 30,30) te.AddEntry te2
You get the idea. I’m pretty sure you’ll know by now how to create the required instances and the associated hierarchy for the “Second Page” and “First Section” entries for the second page of the PDFDocument:
Var teG2 As New PDFTOCEntry(2,"Second Page",0,40) Var teg2a As New PDFTOCEntry(2, "First Section", 100, 100) teG2.AddEntry teG2a
Lastly, all we need to do is add the root instances of the TOC hierarchy (that is te
and teG2
) to the PDFDocument instance itself. So, let’s guess that our PDFDocument instance is pointed by the d
variable. In that case, these two lines of code will complete the Job:
d.AddTOCEntry te d.AddTOCEntry teG2
TOC entries and PDF Viewer Behavior
PDF Viewer apps have their own rules about how or when to “jump” to the X / Y coordinates associated with the TOC entries. In brief:
- If the target coordinates for the target page are already in the view area, then you won’t notice anything. After all you are already seeying the contents of the page that the X / Y coordinates are pointing to! For example, this may be the case when you set the PDF Viewer app to display the two pages of the PDF document at once in the view.
- If the target coordinates for the target page are out of the view area, then the PDF viewer will jump to the specified coordinates putting its contents in the view. The behavior in this case depends on the zoom factor you may be using for displaying the page. That is, it is possible you can see the how the PDF viewer “jumps” to the second page when you click on the “Second Page” entry in the TOC, putting its contents in the view area, but not if you click on the “First Section” or “First Sub-Section” entries for the first page in the document if that page is already in the view area and the zoom is set to “Fit Contents to Window”.
Summary
As we have seen, it is quite simple to create a Table of Contents index for your Xojo PDF documents, adding as many entries as the structure of your PDFs need, in order to provide a better document navigation! Read more about PDFDocument in the Xojo Documentation.
Paul learned to program in BASIC at age 13 and has programmed in more languages than he remembers, with Xojo being an obvious favorite. When not working on Xojo, you can find him talking about retrocomputing at Goto 10 and on Mastodon @lefebvre@hachyderm.io.