If you are learning Object-Oriented Programming (OOP) or are curious about software development, you have probably heard the term Interfaces. This article explains the concept of Interfaces in OOP, how they work, and provides two code examples.
What is an Interface?
An Interface in Object-Oriented Programming acts as a contract or a blueprint. It defines a set of methods (functions) that a class must implement, but it does not provide the actual implementation of those methods. Think of it as a list of rules or requirements that a class agrees to follow. This approach makes your code more flexible, reusable, and easier to maintain.
For example, imagine you are building a game with different types of characters, such as warriors, wizards, and archers. Each character might have an attack method, but the way they attack is different. An Interface ensures that all characters have an attack method, but each class can implement it in its own unique way.
Why Use Interfaces?
- Flexibility: Interfaces allow different classes to be used interchangeably if they implement the same Interface. This makes your code adaptable and scalable.
- Reusability: You can write code that works with any class that implements the Interface, promoting code reuse.
- Consistency: Interfaces enforce consistency across classes, ensuring they all have the required methods.
- Polymorphism: Interfaces enable polymorphism, allowing you to handle different objects through the same interface.
How to Use Interfaces in Xojo
In Xojo, you can create and implement Interfaces to define a set of methods that multiple classes can share, even if they do not share a common superclass. Follow these steps to use Interfaces in Xojo:
- Create an Interface: Go to the Insert menu in the Xojo IDE and select Class Interface. Give the Interface a descriptive name.
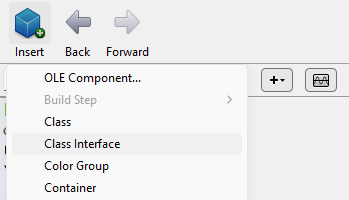
- Define Methods: Add methods to the Interface. These methods have no code; they only define the method names and parameters.
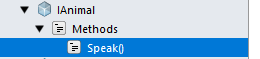
- Implement the Interface in a Class: For a class to use the Interface, select the class and from the Inspector, press the Choose button and mark the newly created Interface.
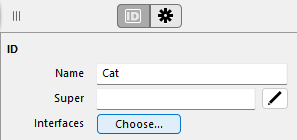
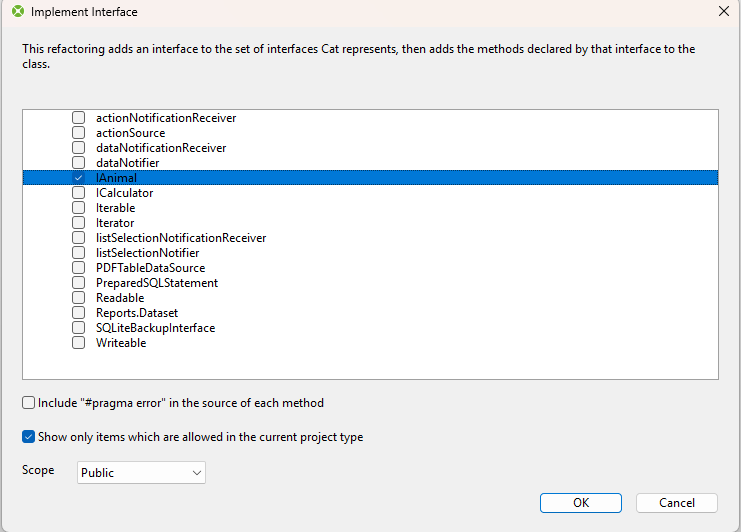
Example 1: Beginner-Friendly Interface
Let’s start with a simple example. Create an Interface called IAnimal
that defines a Speak
method. Then, create two classes, Dog
and Cat
, that implement this Interface.
Step-by-Step Guide
- Define the Interface
- From the Insert menu, select Class Interface, name it
IAnimal
and then create a new methodSpeak
.
- From the Insert menu, select Class Interface, name it
- Create the Dog Class
- Create a class named
Dog
(Insert menu > Class) and from the Inspector, make sure to select the Interface. Xojo will automatically add theSpeak
method to the class so let’s add some code:MessageBox("Woof!")
- Create a class named
- Create the Cat Class
- Create another class, named
Cat
(Insert menu > Class) and add the following code to theSpeak
method:MessageBox("Meow!")
- Create another class, named
- Test the Interface
Add the following code, to test the Interface, either in the Pressed
event of a DesktopButton, or to the Opening
event of a DesktopWindow:
Var animals() As IAnimal
animals.Add(New Dog)
animals.Add(New Cat)
For Each animal As IAnimal In animals
animal.Speak()
Next
Example 2: Interface with Parameters
Now, create a new Class Interface called ICalculator
that defines a Calculate
method. This method takes two numbers as parameters and returns a result. Then, create two classes, Adder
and Multiplier
, that implement this Interface.
Step-by-Step Guide
- Define the Interface
- Add the following method to define the Interface:
Public Function Calculate(a As Integer, b As Integer) As Integer
- Add the following method to define the Interface:
- Create the Adder Class
- Add the following code to the
Calculate
function:Return a + b
- Add the following code to the
- Create the Multiplier Class
- Add the following code to the
Calculate
function:Return a * b
- Add the following code to the
- Test the Interface
Var calculators() As ICalculator
calculators.Add(New Adder)
calculators.Add(New Multiplier)
For Each calc As ICalculator In calculators
MessageBox("Result: " + calc.Calculate(7, 2).ToString)
Next
Some Practical Use Cases for Interfaces
Interfaces are versatile and can be used in many situations to make your code more modular and easier to maintain. Here are some practical use cases for Interfaces in Object-Oriented Programming:
1. Data Access Abstraction
Abstract data access using Interfaces. For example, define an IDatabase Interface with methods Connect, Query, and Disconnect. Classes that implement this Interface can interact with different database systems (like MySQL, PostgreSQL, SQLite), allowing you to switch databases without changing your core code.
2. Cross-Platform Development
Abstract platform-specific functionality using Interfaces. For instance, define an IFileSystem Interface with methods ReadFile and WriteFile. Implement this Interface differently for Windows, macOS, and Linux. Your main code can remain the same across platforms.
3. Polymorphism
Enable polymorphism with Interfaces. This lets you process objects differently based on their actual class, but through the same Interface. This is useful when you want to write code that works with any class that implements a particular Interface.
Key Takeaways
- Interfaces define a set of methods that classes must implement.
- They make your code flexible, reusable, and easier to understand.
- Interfaces enforce consistency across different classes.
- They enable polymorphic behavior, allowing you to write generic code.
Conclusion
Whether you’re building a simple application or a complex system, interfaces are a powerful tool in your Object-Oriented Programming toolkit, and Xojo makes them incredibly easy to use. They promote better code organization, flexibility, and reusability. Start experimenting with Interfaces in your projects, and you will see how they can improve your code!
Additional info on Interfaces: https://documentation.xojo.com/getting_started/object-oriented_programming/interfaces.html
If you have questions or want to share your own Interface examples, feel free to start a forum discussion. Happy coding! 🚀
Gabriel is a digital marketing enthusiast who loves coding with Xojo to create cool software tools for any platform. He is always eager to learn and share new ideas!