Recently, I was asked by a client if it would be possible to build language translation functionality into a Xojo-based middleware solution that I had developed for them. The Xojo app obtains product information (including product names, descriptions, and other marketing-related information) from suppliers via a SOAP call, and returns the data in a JSON-encoded response. They wanted to be able to translate the product information, which is provided in English, to other languages (such as French, German, etc). The client wanted something similar to Google Translate. However, they wanted the translation function to be built directly into the app and to be performed “on demand.”
I did some research and found that Amazon provides a service that does exactly what the client was asking for. The service, called Amazon Translate, is available as one of many services that are available through Amazon Web Services.
In this post, I’ll walk you through the process of getting signed up for Amazon Translate, and then share some code that you can use to add language translation to your own Xojo projects. We’ll use the MBS Xojo CURL Plugin, which makes calling the Amazon Translate API easy. But first, let’s learn a little about Amazon Translate.
Amazon Translate
Amazon Translate is an API that can be used to translate text on demand. You send it text in a “source language,” tell the API what that source language is, as well as the “target language” that you want the text translated to. The API responds with the translated text.
Amazon Translate uses machine learning and neural networks that have been designed specifically for language translation. When performing a translation, the service reads the text one sentence at a time, and reads each word individually, to determine the meaning of a sentence. In other words, the service doesn’t just translate words. Instead, it’s acting as an interpreter. This results in translations that are extremely accurate.
In order to use Amazon Translate, you’ll need an AWS account. If you already have an AWS account, log into the account.
Next, you’ll need to create an “Identity and Access Management” (IAM) user. To do so, under Services, select “IAM” (which can be found in the “Security, Identity, & Compliance” category). This will present you with the IAM Dashboard.
Under Groups, create a new group and give it a recognizable name. For example, you might call the group “translate.” As you are creating the group, give it the “TranslateFullAccess” permission.
Next, create an IAM user. Under Users, click the “Add user” button. Give the user a name such as “XojoTranslater.” For the “AWS access type” be sure to select “Programmatic access.” Add the user to the “translate” group that you created above. There’s no need to assign any tags to the user, so you can skip that step.
And finally, create an Access key for the user. Be sure to make note of both the “Access key ID” and “Secret access key” values that are automatically assigned. You’ll need these in order to make API calls.
The MBS Xojo CURL Plugin
If you don’t already have the MBS Xojo CURL Plugin, download it and install it. To install the plugin, simply drag it into the Xojo “Plugins” folder.
The project will work with an unlicensed version of the MBS Xojo CURL Plugin. In this case, you’ll occasionally see a popup message reminding you to purchase a license. However, I highly encourage you to purchase a license. You can license the plugin individually, or as part of the MBS Complete plugin bundle.
The Xojo Example Project
To demonstrate the Amazon Translate service, I’ve put together an example Xojo desktop project (project file). Before running the application, be sure to set the three App-level properties: AWSAccessKeyId, AWSSecretAccessKey, and the AWSRegion. For the AWSAccessKeyId and AWSSecretAccessKey properties, use the values that you set when you created the Access key for the user. For the AWSRegion, refer to this and use the value that makes the most sense for you.
Once you’ve configured the app, run it, and it will look something like this.
The Xojo Code
Let’s take a look at some of the code.
The AWSTranslator class is a subclass of the CURLSMBS class. The class does two important things: It provides access to a list of the languages that are supported, and provides a method for calling the Amazon Translate API.
The “Languages” property is a dictionary that you can use to get the names of the languages that Amazon Translate supports and the corresponding language codes. The example app uses this dictionary to populate the “Translate From” (SourcePopupMenu) and “Translate To” (TargetPopupMenu) popup menus.
The Translate method creates the payload that gets sent to the Amazon Translate API, and sets special HTTP headers that are needed in order to call the API. But most importantly, it uses the CURLSMBS class’s “SetupAWS” method to configure the CURL instance so that all of the steps that are required to “sign” the API request are done automatically. This is an amazingly convenient function of the MBS Xojo CURL Plugin. You can get a sense of what’s involved in signing the request here.
To translate text using the AWSTranslator class, you create an instance, configure it with your IAM credentials and AWS region, pass it the source language text, the source language code, and the target language code. In the example app, this all handled in the Window1.Translate method. The values are pulled from the App-level properties, as well as the various window controls. Finally, you call the AWSTranslator instance’s Translate method. If the translation is successful, the translated text will be available via the TranslatedText property. If any errors occur, the ErrorType and ErrorMessage properties will be set.
The “Estimated Translation Cost” is calculated whenever the Source Text changes. The value is calculated by taking the length (number of characters) of the Source Text and multiplying it by 0.000015 (the average cost for translating a single character).
Wrapping Up
I’ve been amazed at the speed and accuracy of the Amazon Translate service. And after developing the AWSTranslator class, I was able to quickly and easily add language translation to my client’s middleware app.
I hope you’re equally impressed by the Amazon Translate service, and that you find the AWSTranslator class to be helpful in your own Xojo projects.
Tim Dietrich is a custom software developer based in Richmond, Virginia. To learn more, visit: https://timdietrich.me
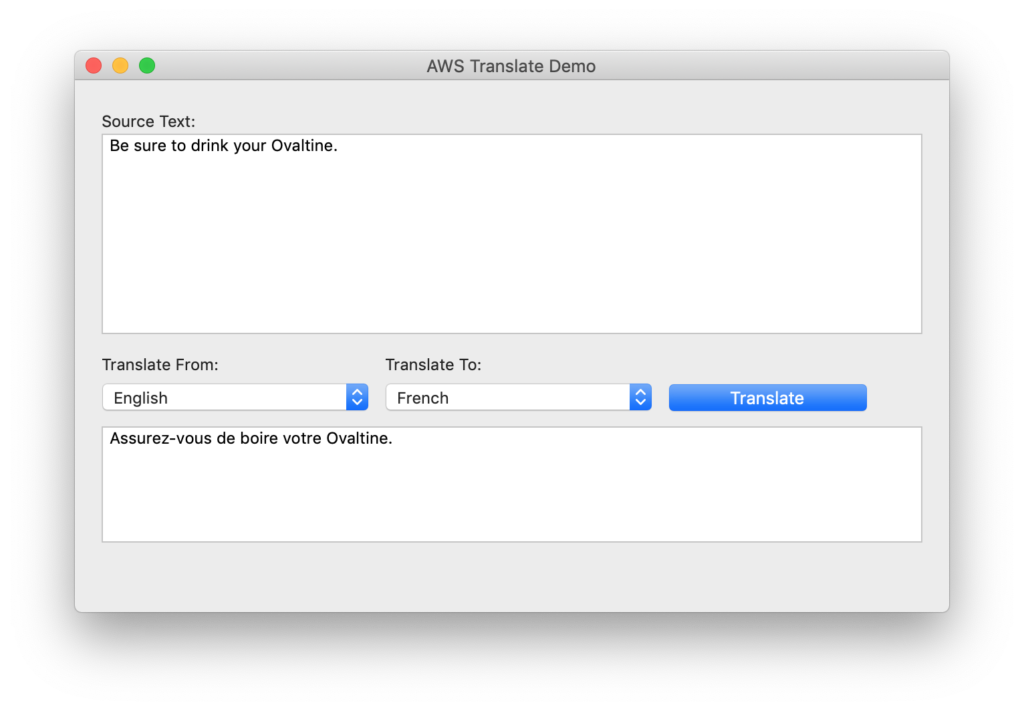