First available on iOS, staring with 2021 Release 1 you can now also use the Rotate, Translate, SaveState and RestoreState methods with Desktop projects.
The Rotate method rotates drawing by the specified angle (in radians). The code below rotates the drawing area 45 degrees and draws a square so it will look like a diamond:
// Rotate the entire drawing area around its center // and draw a rectangle Const Pi = 3.14159 g.Rotate(Pi / 4, g.Width / 2, g.Height / 2) // 45 degrees or 1/8 of a circle g.DrawingColor = Color.Blue g.FillRectangle(g.Width / 2 - 20, g.Height / 2 - 20, 20, 20)
You can use Translate to change the origin point for drawing. Used in combination with Rotate, this code rotates a square around a center point:
// Rotate square in center of graphics area Const Pi = 3.14159 g.Translate(g.Width / 2, g.Height / 2) g.Rotate(Pi / 4) // 45 degrees or 1/8 of a circle g.DrawingColor = Color.Blue g.FillRectangle(10, 10, 50, 50)
With a few tweaks and a Timer, you can animate this (download project):
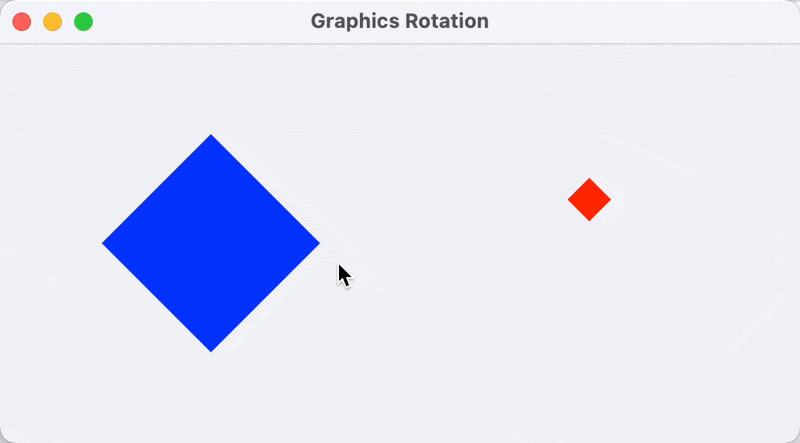
SaveState and RestoreState do what their names imply. They let you save the state of the graphics area so it can be restored later. SaveState can save this information:
- Translate
- Clip region
- PenSize
- AntiAliased
- DrawingColor
- Font
- Underline
- Rotate (rotation)
This code draws a blue rectangle, saves the state and then draws a red rectangle. It then calls RestoreState which puts the color back to blue and draws another blue rectangle:
g.DrawingColor = Color.Blue g.FillRectangle(10, 10, 20, 20) g.SaveState g.DrawingColor = Color.Red g.FillRectangle(50, 50, 20, 20) // Restore to state where FillColor is Blue g.RestoreState g.FillRectangle(10, 50, 20, 20)
You might find that these new methods can serve as an alternative to Object2D for certain types of graphics drawing.
There are also other new Graphics features in 2021r1, such as Scale and Outline, which you can read about on the Graphics doc page.