In this first beta release of Android, there is some simple support for creating Android libraries and calling their methods from Xojo code. The Android libraries have to be written in Kotlin and compiled as AAR library files. Xojo code can call into the library using Declares.
To create an Android library you will need to use Android Studio, which you should already have installed as its compiler toolchain and SDK are required by Xojo.
Create a New Android project (File->New->New Project), choosing “No Activity” from the Phone and Tablet section.
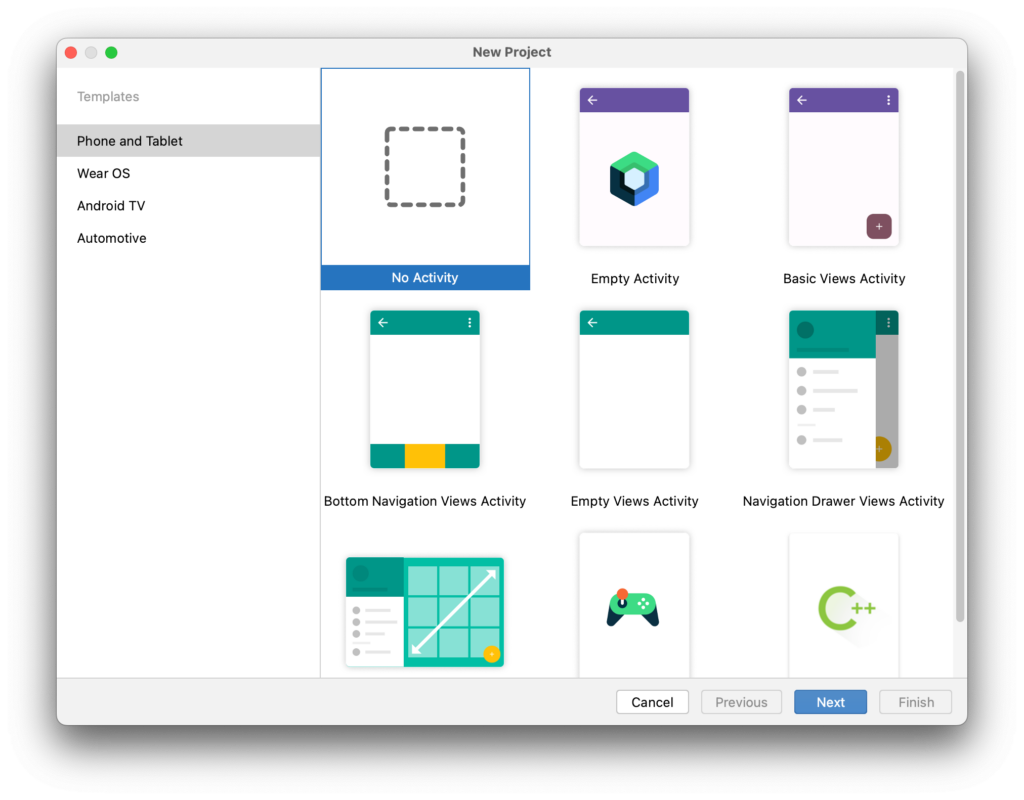
You should be sure to choose Kotlin as the language and the Minimum SDK should be 26 to match what Xojo uses. For this example, I’ve named the project “LibraryTest”.
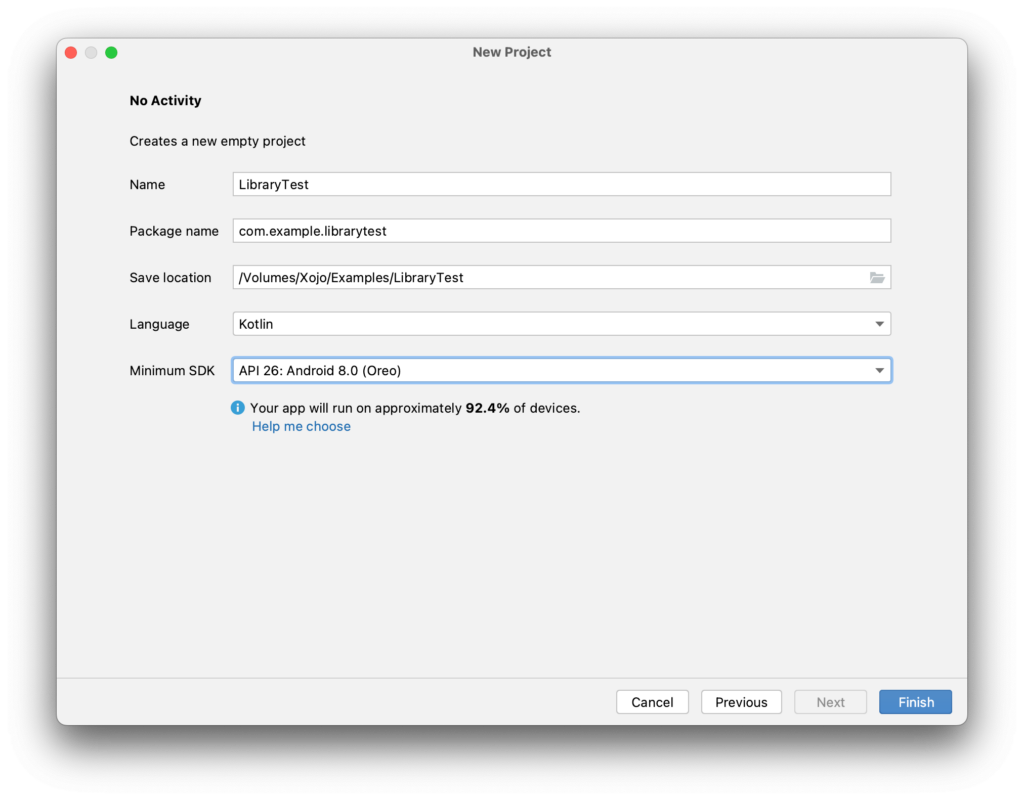
In this project, add a module (File->New->New Module), choosing the “Android Library” template. Here you can name your library, choose the language, etc. Again, stick with Kotlin.
I’m naming the library “utility” so that it can contain a random collection of things.
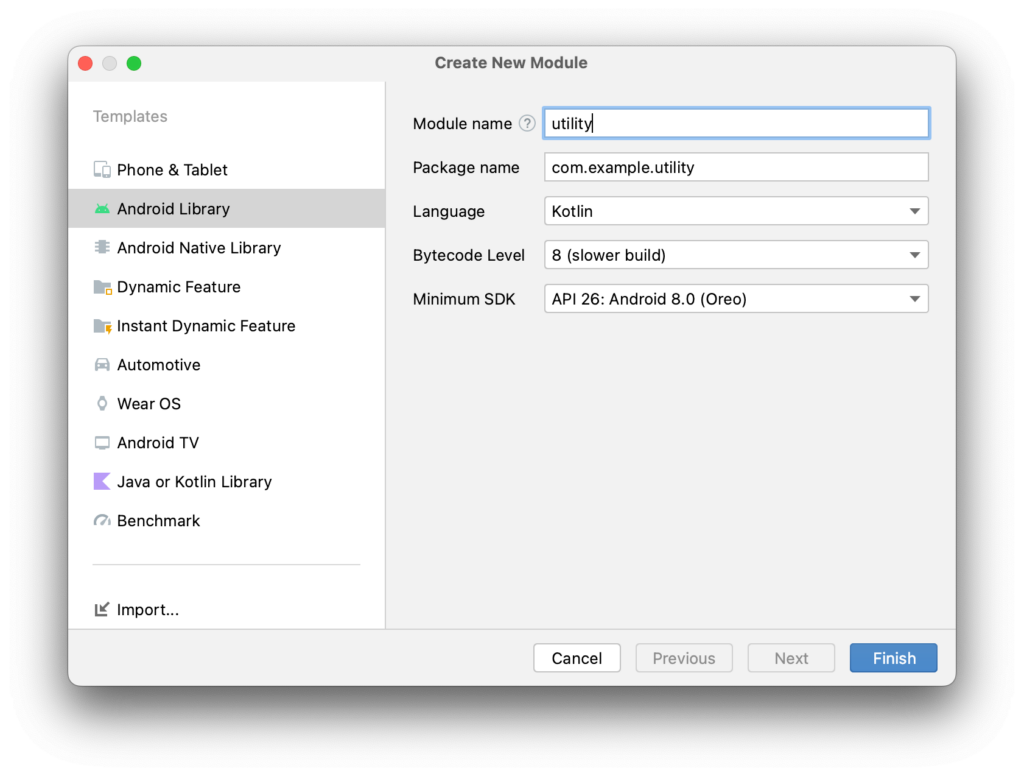
In the Android Studio navigator, expand the utility library and then the java folder. Yes, it is weird that Kotlin code is in a Java folder, but a lot about Android Studio is weird to me.
Right-click on “com.example.utility” and select New->Kotlin Class/File. In the selector, choose “Class” and type the name of the class as “regex” and press return. In this class will be a method to do some simple regex pattern matching.
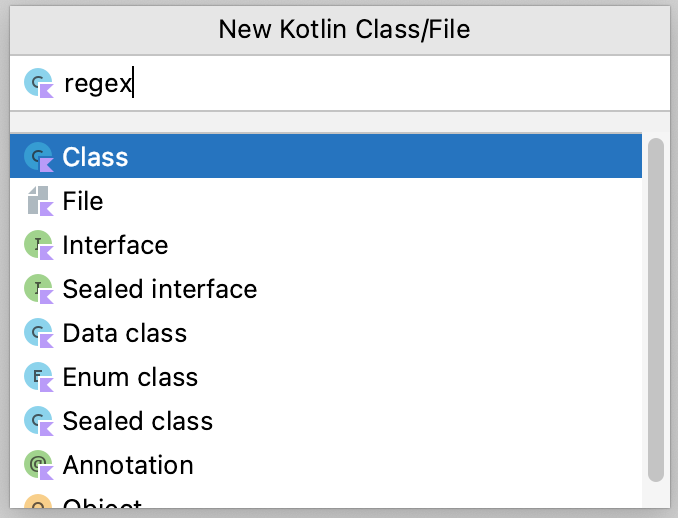
This creates an empty class:
package com.example.utility
class regex {
}
Currently Xojo can only talk to methods in the companion class. These methods are similar to Shared methods in Xojo and work without creating a specific class instance.
In the class, add the companion object so that it looks like this:
package com.example.utility
class regex {
companion object {
}
}
Inside the companion object, add a method that will do simple regex pattern matching. Your code now looks like this:
package com.example.utility
class regex {
companion object {
fun regexmatch(regex: String, text: String): Boolean {
// Use the regex pattern
val pattern = Regex(regex)
// Returns true if it matches, false if it does not.
return pattern.matches(text)
}
}
}
The last step is to change the Kotlin version to match the version Xojo uses. In the Android Studio navigator, open Gradle Scripts and double-click “build.gradle (Project: LibraryTest)” to open it.
Change the last line with the kotlin.android version from whatever is there to “1.6.20”:
// Top-level build file where you can add configuration options common to all sub-projects/modules.
plugins {
id 'com.android.application' version '8.0.2' apply false
id 'com.android.library' version '8.0.2' apply false
id 'org.jetbrains.kotlin.android' version '1.6.20' apply false
}
Note: You may be prompted to do a Gradle sync after making this change. Go ahead and do the sync if prompted.
The last thing to do in Android Studio is to build the library. From the Build menu, choose “Select Build Variant”. In the Build Variants panel that appears, click on the Active Build Variant value for the utility row and change it from debug to release.
Select Build->Rebuild Project to build the library file that Xojo will use.
In your file system, go to your project’s location and fine the aar file, which is located here: LibraryTest/utility/build/outputs/aar/utility-release.aar Make a note of this as we will be using it with Xojo in a moment.
Speaking of Xojo, create a new Xojo Android project and name it LibraryTest. Add a button and a table like so:
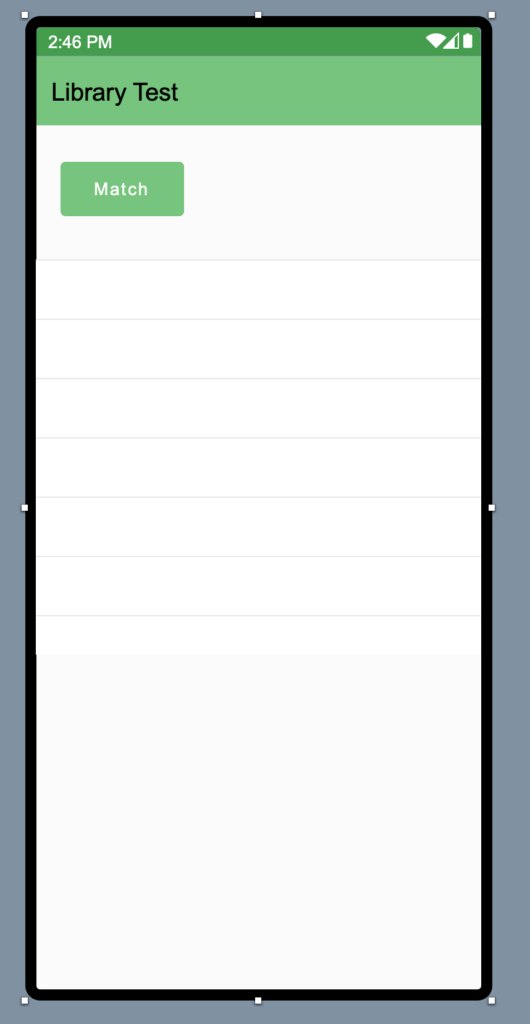
In the button’s pressed event, write the Declare statements to call the regexmatch method in the library, passing it a regex pattern and a string to test. Here is the code, which tests three strings and outputs the result to the table:
Declare Function regexmatch Lib "com.example.utility.regex" (pattern As CString, value As CString) As Boolean
// Look for g, followed by any number of "ee" pairs and ending with ks
Var pattern As String = "g([ee]+)ks?"
Var result As Boolean
Var values() As String = Array("geeks", "geeeeeeeeeeks", "geeksforgeeks")
For Each v As String In values
result = regexmatch(pattern, v)
Table1.AddRow(v, result.ToString)
Next
// From: https://www.geeksforgeeks.org/kotlin-regular-expression/
The important thing to note in this code is the use of CString in place of String, a requirement when working with a Declare even if it doesn’t directly apply to Android.
Also note that you need to provide the full path to the class. You have to use “com.example.utility.regex”, not just “regex”.
The next step is to add the Library to the Xojo project. Select Android in the Build Settings and add a Copy Files Build Step (Insert->Build Step->Copy Files).
Expand Android and click on CopyFiles1. Rename it to LibCopy and drag it so that it is before the Build entry. Change the Destination to “Framework Folder”.
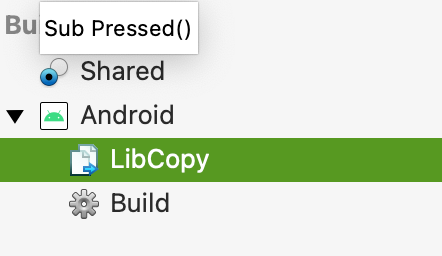
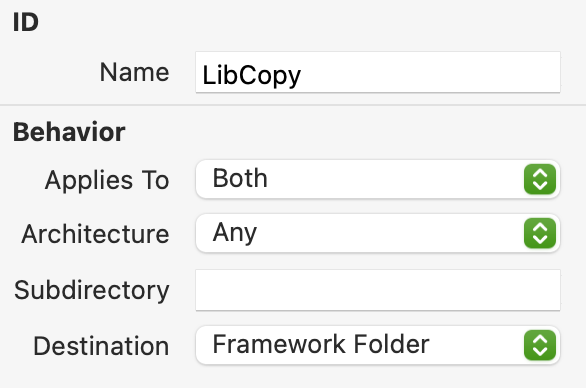
Add the library that we built with Android Studio to this LibCopy step. Click “+” in the command bar and locate utility-release.aar.
Note: If you still have Android Studio open at this point, you should close it, otherwise it will lock debugger access and prevent the Xojo app from starting.
Pro Tip: You can keep Android Studio open and run from Xojo by choosing this preference setting: Build, Execution, Deployment -> Debugger -> Use existing manually managed server. You’ll want to set it back to “Automatically start and manage the server” if you use Android Studio for anything else. Constantly flipping that setting is common when working with Android libraries.
Run the project. If you did everything correctly, it should launch on your device or emulator. Click the button to see the output which should look like this:
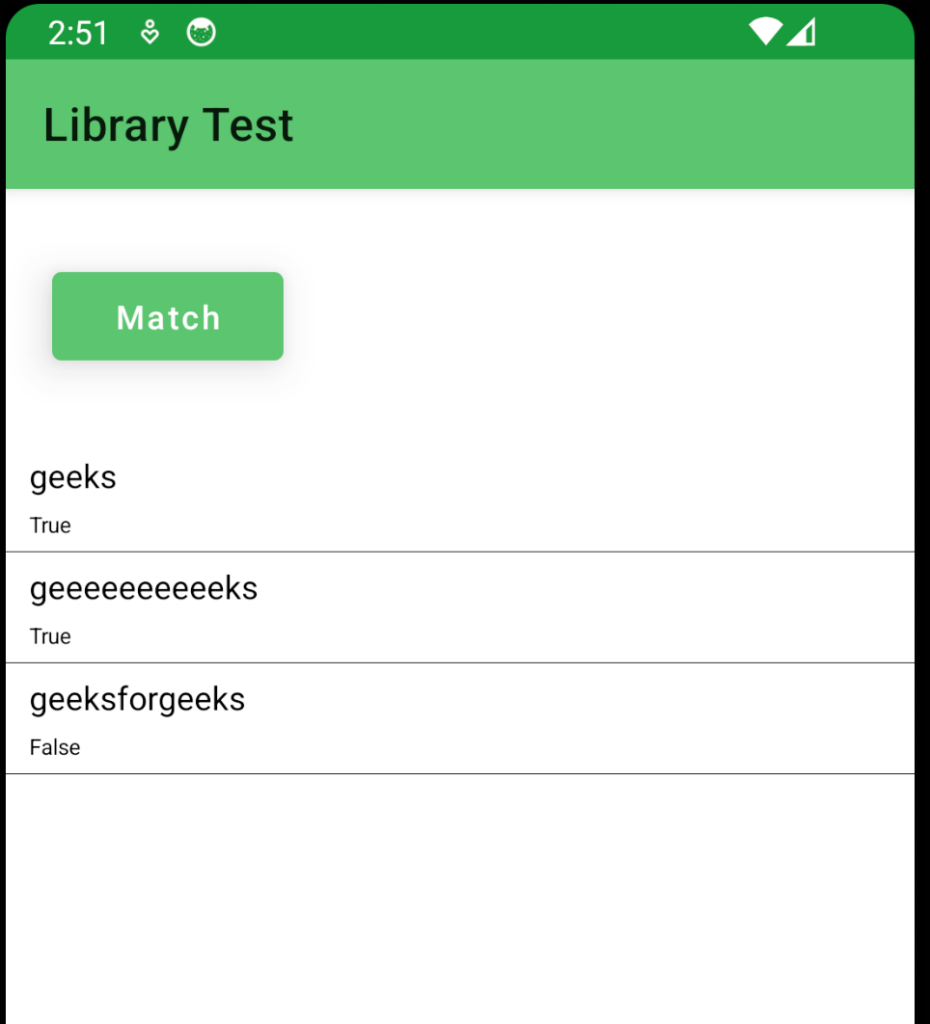
With libraries you can add your own extensions to Xojo’s Android support. You are limited to simple types at the moment, but that still opens up a lot of capabilities. This capability will continue to be expanded through the Android beta.
Feel free to share any Kotlin libraries you create in the Android topic of the forum.
Download this Example RegEx Library
Here is another simple Library you can download and examine.
Paul learned to program in BASIC at age 13 and has programmed in more languages than he remembers, with Xojo being an obvious favorite. When not working on Xojo, you can find him talking about retrocomputing at Goto 10 and on Mastodon @lefebvre@hachyderm.io.