Application programming interfaces (APIs) are essential for enabling digital interactions. They facilitate communication and data sharing between systems, forming the backbone of many applications and services.
This article examines Xojo’s capabilities by building a simple web API service. You will learn to handle different HTTP methods, manage requests, and send meaningful responses. Xojo’s simplicity makes it an excellent choice for experienced developers transitioning from other languages, as well as those familiar with its user-friendly language.
Foundation: The HandleURL Function
At the heart of every Xojo-developed web API service is the HandleURL function, included in the App class, responsible for handling the request-response cycle. It receives incoming web requests (WebRequest), processes them based on their characteristics, and crafts appropriate responses (WebResponse) to send back.
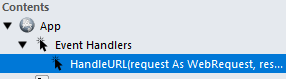
Defining API Endpoints for GET Requests
GET requests are primarily used to retrieve data. To handle these requests effectively, let’s see how to define API endpoints that correspond to specific data or actions in the following code example:
If request.Method = "GET" Then
Select Case request.Path
Case "time"
Var currentTime As String = DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss")
response.Write("{""message"": """ + currentTime + """}")
response.Status = 200
Return True
Case "hello"
response.Write("{""message"": ""Hello, World!""}")
response.Status = 200
Return True
End Select
End If
Here, we define two endpoints: /time and /hello.
A GET request to /time will return the current date and time in JSON format, while a request to /hello will return a friendly “Hello, World!” greeting.
The response.Status is set to 200, indicating a successful response. Learn more here about HTTP status codes.
Defining API Endpoints for POST Requests
While GET requests primarily retrieve data, POST requests submit data to the API for processing. Consider submitting a form online – you are sending data to the server via a POST request.
If request.Method = "POST" Then
Select Case request.Path
Case "some-data"
Try
Var jReceivedData As New JSONItem(request.Body)
Response.Write(jReceivedData.ToString)
Response.Status = 200
Return True
Catch e As JSONException
response.Write("{""error"": ""Internal Server Error""}")
response.Status = 500
Return True
End Try
End Select
End If
In this example, a POST request to the /some-data endpoint expects to receive JSON data (request.Body) for processing.
If the data is successfully parsed, the API responds with the received data.
Otherwise, an error status and message are returned to the client.
Bringing it all Together
Now that you know how to manage “GET” and “POST” requests, you can start building more complex APIs, with different endpoints available for different methods. To further illustrate API endpoint development, consider the following detailed code example that can be used as template:
Function HandleURL(request As WebRequest, response As WebResponse) Handles HandleURL as Boolean
response.MIMEType = "application/json"
Try
Select Case request.Method
Case "GET"
Select Case request.Path
Case "time"
Var currentTime As String = DateTime.Now.ToString("yyyy-MM-dd HH:mm:ss")
response.Write("{""message"": """ + currentTime + """}")
response.Status = 200
Return True
Case "hello"
response.Write("{""message"": ""Hello, World!""}")
response.Status = 200
Return True
Else
response.Write("{""error"": ""Not Found""}")
response.Status = 404
Return True
End Select
Case "POST"
Select Case request.Path
Case "some-data"
Try
Var jReceivedData As New JSONItem(request.Body)
Response.Write(jReceivedData.ToString)
Response.Status = 200
Return True
Catch e As JSONException
response.Write("{""error"": ""Internal Server Error""}")
response.Status = 500
Return True
End Try
Else
response.Write("{""error"": ""Not Found""}")
response.Status = 404
Return True
End Select
End Select
Catch e As RuntimeException
response.Write("{""error"": ""Internal Server Error""}")
response.Status = 500
Return True
End Try
response.Write("{""error"": ""Not Found""}")
response.Status = 404
Return True
End Function
Small note: observe the start line within the HandleURL function.
response.MIMEType = "application/json"
This line of code informs the client (browser, application) that the API service is responding using the JSON format. Setting the MIMEType to “application/json” is best practice and helps prevent any potential parsing errors on the client side.
Next Steps
You might notice that some code is repeating itself (hint: the error responses), so a good idea would be to create a helper method that deals with this particular type of code repetition. Additionally, consider moving the code from the HandleURL function to a separate method that is then invoked within the HandleURL function for improved organization. This restructuring will enhance code organization, making it easier to maintain, read, and improve the efficiency of the program.
P.S.: You should also check the article about microservices and their purpose to get a better idea of what kind of API interfaces you can create.
Gabriel is a digital marketing enthusiast who loves coding with Xojo to create cool software tools for any platform. He is always eager to learn and share new ideas!