Android has been updated to support several standard Xojo language features, including:
- Structures
- ByRef parameters
- Operator_Lookup
- Operator_Convert
Structures
Structures are a fixed-size data structure that can contain properties of simple types, such as Integer, String or Boolean.
Normally you would only use Structures with Declare API calls, but they can also work as a lightweight, project item specific data structure.
For Android, Structures are not fixed-sized data structures and are not appropriate for Declares, but they offer slightly more flexibility, especially with regard to Strings. When you add a String to an Android Structure, the specified size is ignored; you can store any size string in the property.
Usage is the same. Create a Structure in the Structure editor for the project item, adding the properties you want.
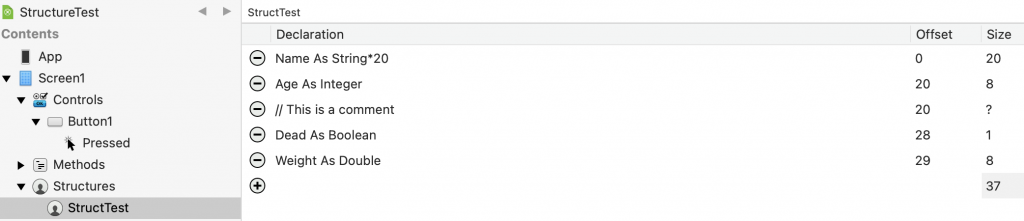
Note that the Offset and Size columns are not relevant in Android since the Structure is not fixed-size.
In code, you create a structure by name:
Var st As StructTest
st.Age = 42
st.Dead = True
st.Name = "Bob Roberts"
st.Weight = 200.6
Since Android Structures are not size-based, the ByteValue, Size and StringValue members are not useful and will raise an UnsupportedOperationException when used.
ByRef Parameters
ByRef parameters allow you to pass a parameter “by reference” so that the caller can access its new value if it was changed. By default, parameters are passed ByVal (or “by value”) so that the caller does not see any changes made to them in the method.
Use the ByRef keyword in front of the parameter to make it ByRef.
The ByRef page in the docs has more information.
Operator_Lookup
Operator_Lookup might be unique to Xojo. When you add an Operator_Lookup method to a class, it essentially tells the compiler to not do a syntax check on method or property names. Instead, if the name that follows the “.” is not an actual member of the class, then the name is passed as a string parameter to the Operator_Convert method.
You can do some strange things with Operator_Lookup, including making your code much more difficult to debug. Use this advanced feature wisely.
Learn more about Operator_Lookup in the docs.
Operator_Convert
Operator_Convert is used with classes to allow them to either construct a new instance from a value of a different type or to allow them to return a version of itself that is compatible with the destination type.
Here is an example of a Person class that uses Operator_Lookup. The Person class has a property: FullName As String. This is how you might normally create an instance and assign a value to FullName:
// Person is a class with a property FullName As String
Var p1 As New Person
p1.FullName = "Bob Roberts"
Now add an Operator_Convert method to the class like this:
Sub Operator_Convert(name As String)
Self.FullName = name
End Sub
With that method, you can now assign a string value to a Person variable and it will create an instance and assign the string value to the FullName property. The syntax looks like this:
Var p As Person
p = "Bob Roberts"
You can also add an Operator_Convert method that returns a representation of a class instance as a different type. For example, say you wanted a Person instance to be able to convert itself to a string. First you have to decide what the string would be and in this case it makes sense to use FullName.
Here is that Operator_Convert for Person:
Function Operator_Convert As String
Return Self.FullName
End Function
This code then assigns a Person instance to a Label’s text property where it gets assigned what is in the FullName property:
Var p2 As New Person
p2.FullName = "Janet Jane"
Label1.Text = p2
Like with Operator_Lookup, Operator_Convert is an advanced feature. Use it carefully. It certainly allows for some code to be simplified, but it also means that code might not be as clear as it would if it used more standard syntax.
Paul learned to program in BASIC at age 13 and has programmed in more languages than he remembers, with Xojo being an obvious favorite. When not working on Xojo, you can find him talking about retrocomputing at Goto 10 and on Mastodon @lefebvre@hachyderm.io.