Here’s a fun little project you can try to get a feel for simple animation in your iOS apps. As you can see, this project moves a small “ball” around the screen and it bounces off the edges.
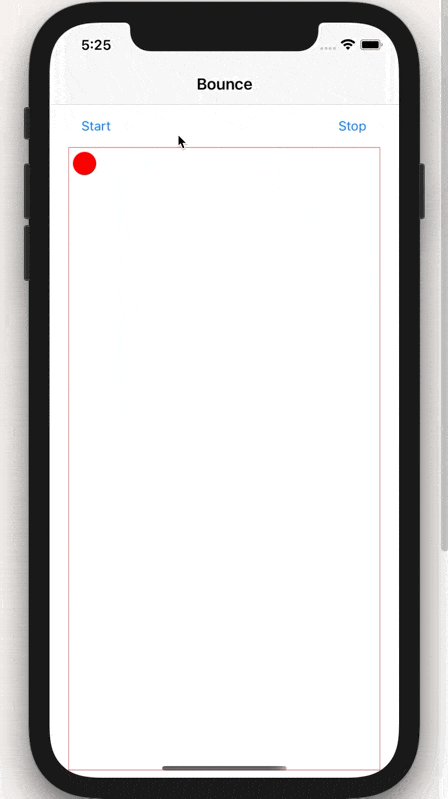
Create a new iOS project and on Screen1 add a couple Buttons and a Canvas, the buttons at the top and the Canvas using up the rest of the space. Also add a Timer.
Add some properties to track the ball’s position and direction:
- Public Property XDirection as Integer = 10
- Public Property XPosition as Integer = 10
- Public Property YDirection as Integer = 10
- Public Property YPosition as Integer = 10
In the Opening event for Screen1, initialize the Timer (which I’ve named MoveTimer):
MoveTimer.Period = 50 MoveTimer.RunMode = Timer.RunModes.Multiple MoveTimer.Enabled = False
Speaking of the Timer, in its Run event put this code to move the ball:
XPosition = XPosition + XDirection YPosition = YPosition + YDirection BounceCanvas.Refresh
Add the Paint and PointerDown events to the Canvas. In the Paint event draw the ball with this code:
Var size As Integer = 25 g.DrawingColor = Color.RGB(255, 0, 0) g.DrawRectangle(0, 0, g.Width, g.Height) g.FillOval(XPosition, YPosition, size, size) If (XPosition + size) > g.Width Then XDirection = -XDirection End If If ( YPosition + size) > g.Height Then YDirection = -YDirection End If If XPosition < 0 Then XDirection = -XDirection End If If YPosition < 0 Then YDirection = -YDirection End If
And in the PointerDown event, reset the ball’s position to the touch:
XPosition = position.X YPosition = position.Y XDirection = -XDirection YDirection = -YDirection
Lastly you can add the Pressed event to the Start button and tell it to start the Timer:
MoveTimer.Enabled = True
Similarly add the Pressed event to the Stop button and tell it to stop the Timer:
MoveTimer.Enabled = False
That’s it. Run the project, click start to watch the ball bounce. Tap on the screen to change its position and direction. You could probably use this as the start of a Pong game!
Oh, and I forgot to mention that the code above was actually copied from the Android project where I first created this. The exact code copied over to iOS and worked as is with no changes. For a little teaser, here’s Bounce running on Android:
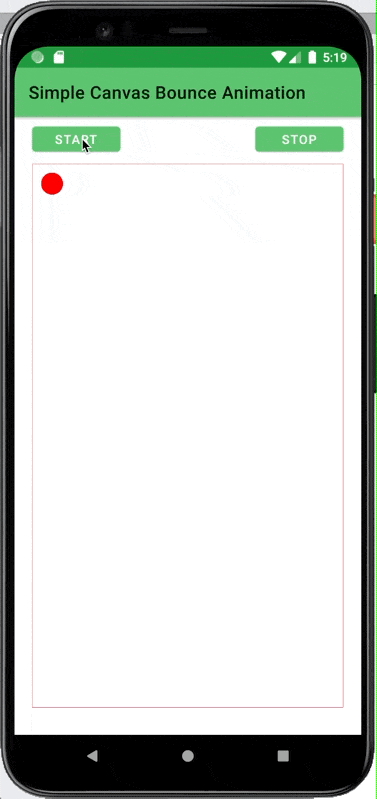