The Game of Life, also known simply as Life, is a cellular automaton devised by the British mathematician John Horton Conway in 1970. You’ve probably seen this around in some form or another, but I ran across it again recently and thought it would be fun to implement in Xojo.
The way it works is that you have a grid of cells. The cells can turn from alive (on) to dead (off) depending on some simple rules:
- Any live cell with two or three live neighbors survives.
- Any dead cell with three live neighbors becomes a live cell.
- All other live cells die in the next generation. Similarly, all other dead cells stay dead.
You start a game of life with your seed pattern and then see how it progress through multiple generations. Here is how the pattern “The R-pentomino” looks:
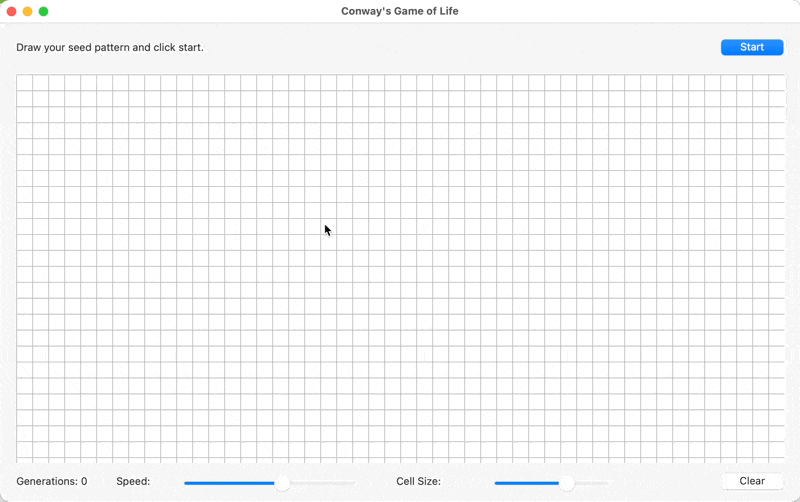
To use Life, draw a pattern in the grid using the mouse. There are several sample patterns on the Game of Life Wikipedia page. After drawing the pattern, click Start.
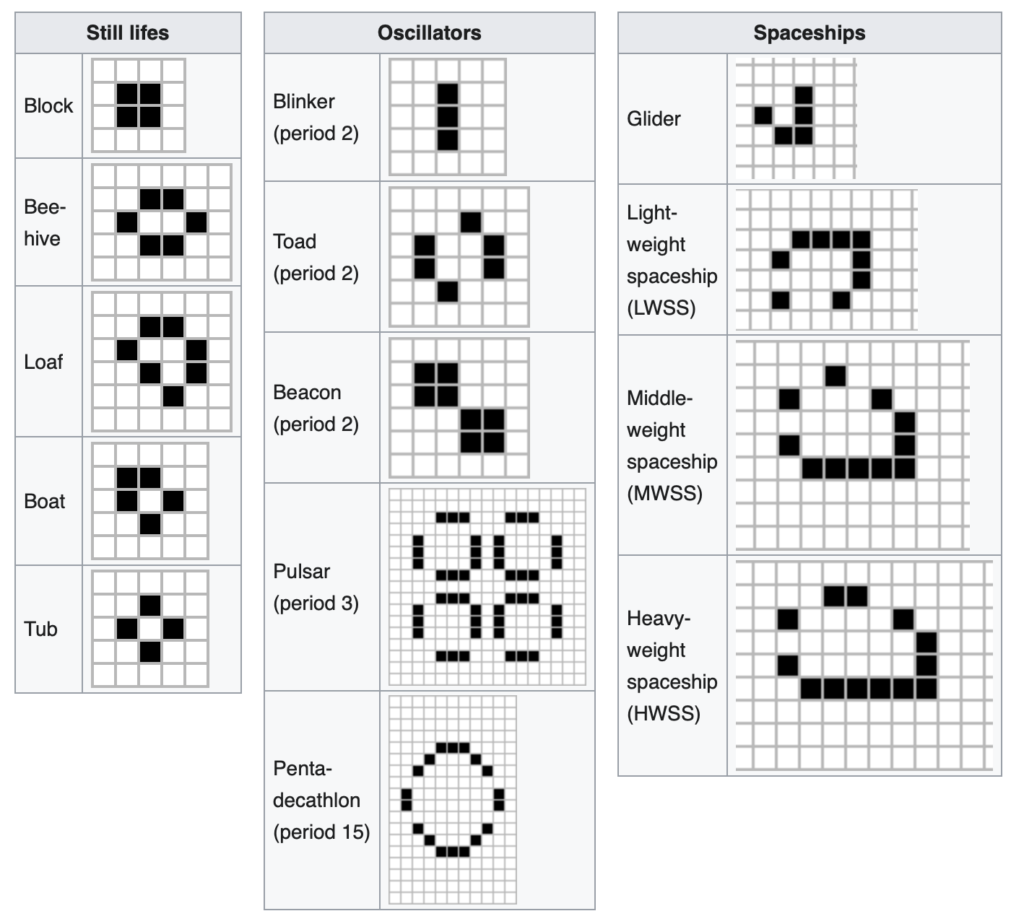
The Xojo project tracks the cells in a two-dimensional array and applies the rules to the array, creating a new array that is then drawn in a Canvas.
The primary method is called Life() and looks like this:
Public Sub Life() // Loop through all the cells and apply these rules. // https://en.wikipedia.org/wiki/Conway%27s_Game_of_Life // 1. Any live cell With two Or three live neighbours survives. // 2. Any dead cell With three live neighbours becomes a live cell. // 3. All other live cells die In the Next generation. Similarly, // all other dead cells stay dead. // Start with a new blank cell grid and apply rules to it // based on the existing cell grid Var newCells(1, 1) As Boolean Var xMax As Integer = Cells.LastIndex(1) Var yMax As Integer = Cells.LastIndex(2) newCells.ResizeTo(xMax, yMax) For x As Integer = 0 To Cells.LastIndex(1) For y As Integer = 0 To Cells.LastIndex(2) Var neighborCount As Integer = CountNeighbors(x, y) If Cells(x, y) = True And (neighborCount = 2 Or neighborCount = 3) Then newCells(x, y) = True ElseIf Cells(x, y) = False And neighborCount = 3 Then newCells(x, y) = True End If Next Next GenerationCount = GenerationCount + 1 Cells = newCells End Sub
You can download the project here:
Although this is a desktop project, it should be easily adaptable to other targets. I love to see what improvements the clever folks in the Xojo community can do. Some ideas include: use lots of colors for drawing, save a drawing to a file, reload a drawing from a file as a staring point. What enhancements would you like?
Share your modifications in the forum!