Xojo’s implementation of OpenGL maps directly to the OpenGL API. This means you can often use OpenGL tutorials on the internet with Xojo with almost no code changes.
I found a tutorial on creating a rotating cube and with some minor help from StackOverflow, tweaked it to display a rotating cube that moves around in a mesmerizing way.
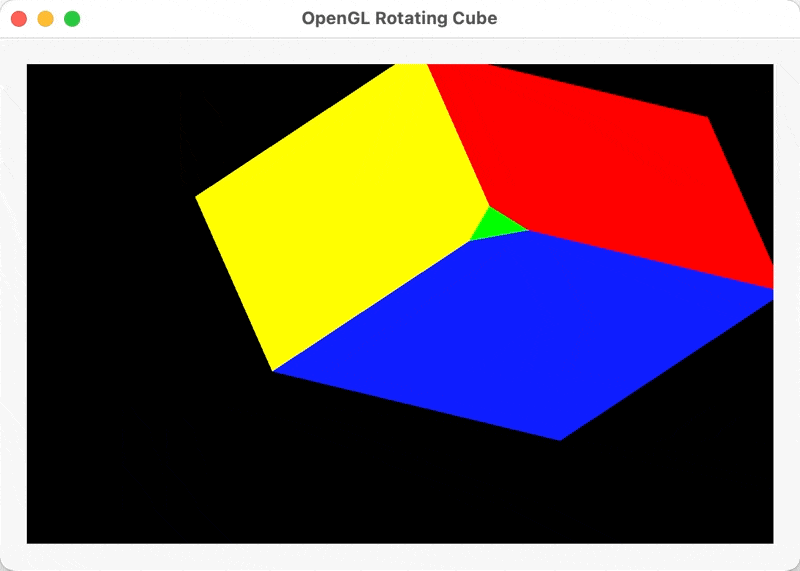
The main code for this is in the DrawCube() method that is called by the Render event of the OpenGLSurface:
OpenGL.glClear(OpenGL.GL_COLOR_BUFFER_BIT Or OpenGL.GL_DEPTH_BUFFER_BIT) OpenGL.glLoadIdentity OpenGL.glScalef(0.5, 0.5, 0.5) // Rotate the cube OpenGL.glRotatef(xRot, 1, 0, 0) OpenGL.glRotatef(yRot, 0, 1, 0) OpenGL.glRotatef(zRot, 0, 0, 1) // Move it around the z axis OpenGL.glTranslatef(0, 0, z) OpenGL.glBegin(OpenGL.GL_QUADS) // Draw the cube OpenGL.glColor3f(1.0, 1.0, 0.0) // Yellow OpenGL.glVertex3f(1.0, 1.0,-1.0) OpenGL.glVertex3f(-1.0, 1.0,-1.0) OpenGL.glVertex3f(-1.0, 1.0, 1.0) OpenGL.glVertex3f( 1.0, 1.0, 1.0) OpenGL.glColor3f(1.0, 0.0, 1.0) // Magenta OpenGL.glVertex3f( 1.0, -1.0, 1.0) OpenGL.glVertex3f(-1.0, -1.0, 1.0) OpenGL.glVertex3f(-1.0, -1.0, -1.0) OpenGL.glVertex3f( 1.0, -1.0, -1.0) OpenGL.glColor3f(1.0, 1.0, 1.0) // White OpenGL.glVertex3f( 1.0, 1.0, 1.0) OpenGL.glVertex3f(-1.0, 1.0, 1.0) OpenGL.glVertex3f(-1.0,-1.0, 1.0) OpenGL.glVertex3f( 1.0,-1.0, 1.0) OpenGL.glColor3f(1.0, 0.0, 0.0) // Red OpenGL.glVertex3f( 1.0, -1.0, -1.0) OpenGL.glVertex3f(-1.0, -1.0, -1.0) OpenGL.glVertex3f(-1.0, 1.0, -1.0) OpenGL.glVertex3f( 1.0, 1.0, -1.0) OpenGL.glColor3f(0.0, 0.0, 1.0) // Blue OpenGL.glVertex3f(-1.0, 1.0, 1.0) OpenGL.glVertex3f(-1.0, 1.0, -1.0) OpenGL.glVertex3f(-1.0, -1.0, -1.0) OpenGL.glVertex3f(-1.0, -1.0, 1.0) OpenGL.glColor3f(0.0, 1.0, 0.0) // Green OpenGL.glVertex3f( 1.0, 1.0, -1.0) OpenGL.glVertex3f( 1.0, 1.0, 1.0) OpenGL.glVertex3f( 1.0, -1.0, 1.0) OpenGL.glVertex3f( 1.0, -1.0, -1.0) OpenGL.glEnd()
The code does some initialization, scales things to half the size of the viewable area, applies rotations and a translation to get the cube to move about and then draws the cube. A timer adjusts the values for the rotations and translation, telling the cube to re-render itself.
Download the project and play around with it. You might want to try having multiple rotating cubes at once.
Paul learned to program in BASIC at age 13 and has programmed in more languages than he remembers, with Xojo being an obvious favorite. When not working on Xojo, you can find him talking about retrocomputing at Goto 10 and on Mastodon @lefebvre@hachyderm.io.