The annotations feature on PDFDocument has been significantly extended in the release of Xojo 2022r2. Continue reading to learn about the new annotation types.
Currently, Xojo’s PDFDocument supports the most common type of annotations for PDF – you know those little yellow boxes displayed like sticky notes on the pages of the PDF document that display their contents when clicked on.
Now with Xojo 2022r2 and later you have more options! And while most of these don’t look like “real” annotations or like something made using the Graphics methods, they will behave as the annotations you are familiar with using in PDF. What I mean by this is that they will display the associated text for the annotation while also allowing the user to Accept/Decline the annotation or interact with them by adding, for example, new replies.
Before reviewing the new options, let me just remind you: The PDF Standard dictates how some features must be implemented for a PDF. After that, it is up to the developers of the PDF viewer app to decide what features are implemented, keep in mind results can vary depending on the PDF viewer you or your user chose to use. As other many things related with PDF files, don’t expect every PDF viewer apps out there to support or adhere to the annotations outside of the most basic ones.
Adobe is the original creator of the PDF specification and its free PDF viewer, Acrobat Reader app does support everything Xojo’s PDFDocument offers.
Attaching Files to the PDF
You’ll be able to embed any kind of file (except for those based in a Bundle structure) into the PDF document itself, for example an Excel file, a Hi-Res image, etc. Then the user viewing the PDF document will be able to open the attached file clicking in the annotation using whichever PDF Viewer app their computer/device has installed for the attached file format. Because this is an annotation, the user will be able to also edit the annotation or add new replies to it.
This is an example about how to create these kind of annotations:
Var d As New PDFDocument
Var g As Graphics = d.Graphics
Const kContentText As String = "Attached File Annotation: double click on me!"
g.FontSize = 20
g.DrawText(kContentText, 40, 60)
If exampleFile <> Nil Then
// Creating a new EmbeddedSound.
d.AddEmbeddedFile(exampleFile, 40, 60, g.TextWidth(kContentText), 20)
// Saving the created PDF document to the selected path.
Var f As FolderItem = FolderItem.ShowSaveFileDialog("","PDFAttachedFile.pdf")
If f <> Nil Then
d.Save(f)
End If
End If
Here exampleFile
is a FolderItem property that has been added to the project and that’s pointing to the file to embed.
And this is how it looks like in the resulting PDF file:
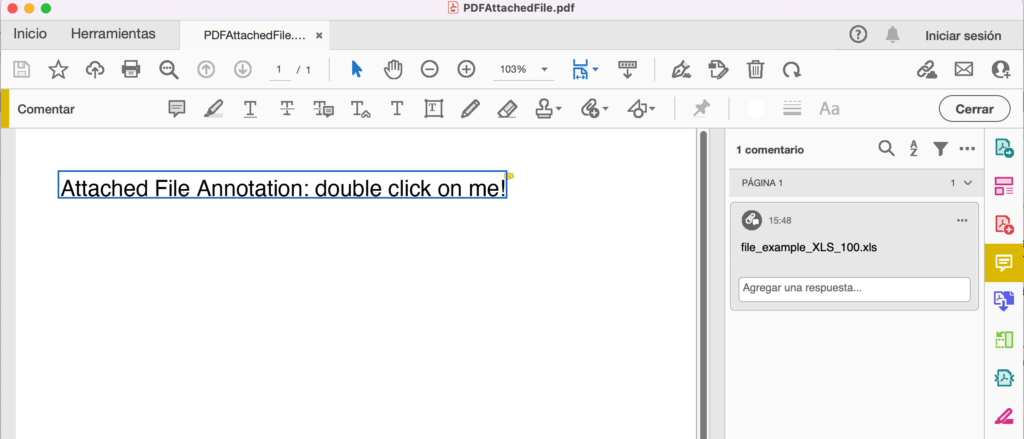
Callout Annotations
These kind of annotations are perfect for pointing our or calling attention to any other item in the PDF page. They are highly customizable- you can decide for example a solid or dashed line, the termination of the line, the color and, of course the text to be displayed in the annotation itself.
This is an example showing how to add a Callout annotation to a PDFDocument:
Var d As New PDFDocument
Var g As Graphics = d.Graphics
Const kContentText As String = "Callout Annotation in PDF"
g.FontSize = 40
g.Bold = True
g.DrawText(kContentText, 40, 60)
g.Bold = False
g.FontSize = 12
g.DrawText(kSampleText, 40, 100, 200)
Var originY As Double = (g.TextHeight(kSampleText, 200) / 2)
Var tCallout As New PDFCallout("Callout Example", New Point(250, originY), New Point(300, originY + 50), _
New Point(350, originY + 50), 100, 50)
tCallout.BorderStyle = PDFAnnotation.BorderStyles.Dashed
tCallout.DashPattern = Array(2.0, 2.0)
tCallout.DrawingColor = Color.Orange
tCallout.LineEndingStyle = PDFAnnotation.LineEndingStyles.OpenArrow
tCallout.Text = "Some sample text in the annotation"
tCallout.FontSize = 9.5
d.AddCallout(tCallout)
// Saving the created PDF document to the selected path.
Var f As FolderItem = FolderItem.ShowSaveFileDialog("","PDFCalloutExample.pdf")
If f <> Nil Then
d.Save(f)
End If
Where kSampleText
is a Constant added to the project containing some sample text to be put in the page of the PDF.
And this is how the Callout will look like when opening the resulting PDF file in the viewer app:
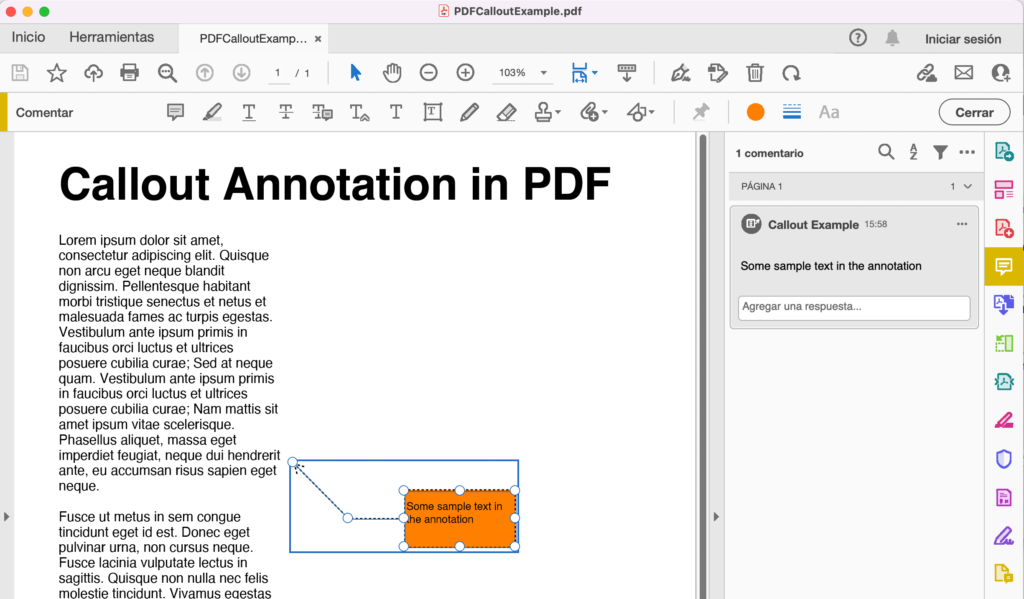
Line Annotations
A “Line” annotation displays a straight line on the page with a text caption inside the line or above it.
It is also possible to add leader lines to both sides of the main line (with possitive or negative values) plus set other properties as the type of the line (solid, dashed, etc), the kind of termination used on both ends of the main line (i.e: open/close arrow, butt, circle, diamond, square…), the drawing color, line width or font size.
This is how a Line annotation looks like when the resulting PDFDocument is viewed using Acrobat Reader (in this case, using Leader lines):
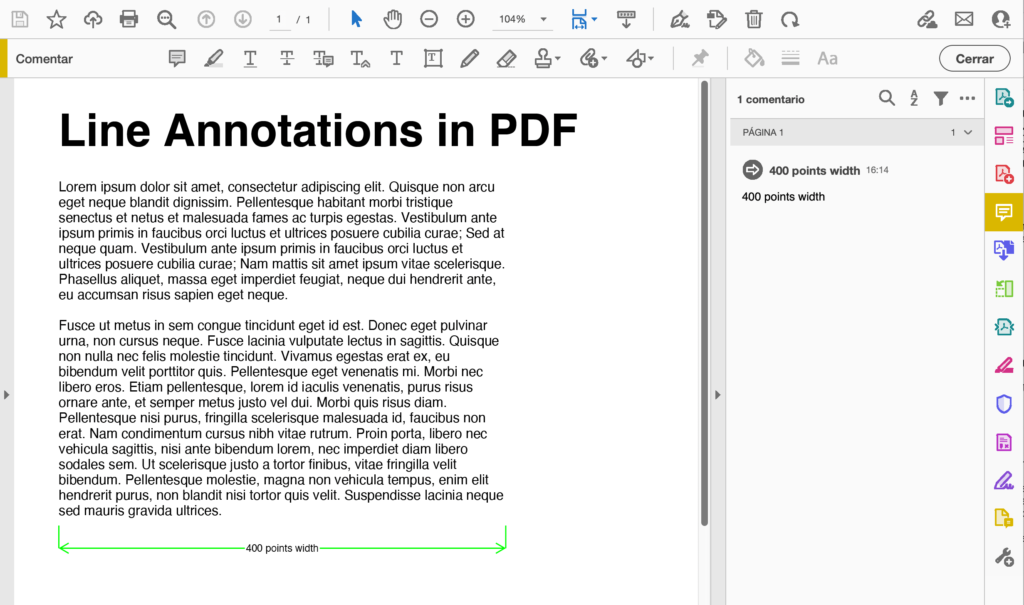
Video Annotations
Yes, you can embed .mp4 files in the PDF created with PDFDocument, and these will be played from the PDF itself. This will increase the size of the generated PDF file because it will add the size of the embedded mp4 file itself! So it is good practice to optimize the video files as much as possible and use the video resolution you want them to be played and not a higher one.
You can also add this kind of content as an Attached File annotation too. In both cases the data of the file will be embedded in the generated PDF file. The main difference in this case is that the mp4 file will be opened using an external app and that you’ll be able to include other video file format besides .mp4.
The way to add this kind of annotation to a PDFDocument instance is quite simple, for example:
Var d As New PDFDocument
Var g As Graphics = d.Graphics
Const kContentText As String = "Embedded Video Annotation"
g.FontSize = 30
g.DrawText(kContentText, 40, 60)
If exampleVideo <> Nil Then
// Creating a new EmbeddedSound.
d.AddEmbeddedMovie(exampleVideo, 40, 100, 478, 360)
// Saving the created PDF document to the selected path.
Var f As FolderItem = FolderItem.ShowSaveFileDialog("","PDFEmbeddedVideo.pdf")
If f <> Nil Then
d.Save(f)
End If
End If
Where exampleVideo
is a FolderItem Property that has been added to the project. This is how a video annotation will look like when opening the resulting PDF in Acrobat Reader:
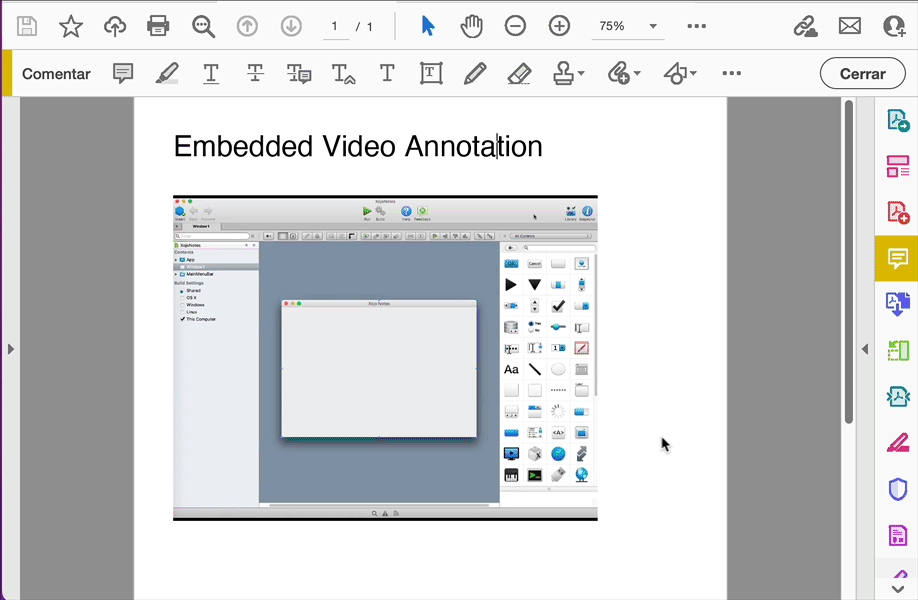
Sound Annotations
In addition to video annotations, it is also possible to add sound files that can be played from the PDF itself. In this case, the audio files need to be in AIFF format with 2 audio channels (stereo), 16 bits of resolution and a sampling frequency of 44,1 kHz.
As it is the case with video annotations, you can opt to add this kind of content as an Attached File annotation, so it will be opened with an external app and opens the range of sound file formats you can embed into the PDF.
The way to add Sound annotations using PDFDocument is shown here:
Var d As New PDFDocument
Var g As Graphics = d.Graphics
g.FontSize = 30
Const kContentText As String = "Embedded Sound Annotation"
Var x, y, width, height As Double
x = g.Width/2 - width/2
y = 60
width = g.TextWidth(kContentText)
height = g.TextHeight
g.DrawText(kContentText, x, y)
If exampleSound <> Nil Then
// Creating a new EmbeddedSound.
d.AddEmbeddedSound(exampleSound,x,y,width,height)
// Saving the created PDF document to the selected path.
Var f As FolderItem = FolderItem.ShowSaveFileDialog("","PDFEmbeddedSound.pdf")
If f <> Nil Then
d.Save(f)
End If
End If
Here exampleSound
is a FolderItem Property added to the project. And this is how a Sound annotation will look when the PDF document is opened using Acrobat Reader:
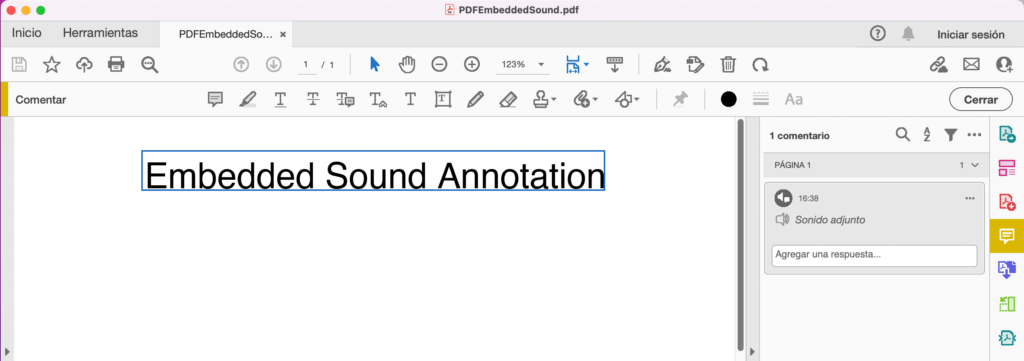
Markup Annotations
A Markup annotation is intended to mark an underlying text content using any of the different available types, for example “Highlight”, “Squiggly” (usually used to mark a text that needs some kind of correction), “Underline” or “Strikeout”. Besides the Markup type, it is also possible to set the color of the annotation.
For example, the following snippet of code adds two Markup annotations to the page of the PDFDocument using the Highlight and Squiggly types:
Var d As New PDFDocument
Var g As Graphics = d.Graphics
Const kContentText As String = "Markup Annotation in PDF"
g.FontSize = 40
g.Bold = True
g.DrawText(kContentText, 40, 60)
// Adding a Markup annotation with the Highlight type (Default)
d.AddMarkup(40,40,g.TextWidth(kContentText),g.FontSize,"Let's focus on this Section")
g.Bold = False
g.FontSize = 12
g.DrawText(kSampleText, 40, 100, g.Width-80)
// Adding a Markup annotation with the Squiggly type
d.AddMarkup(40,195,210,g.FontSize,"Some text to correct!",Color.Red,PDFAnnotation.MarkupTypes.Squiggly)
// Saving the created PDF document to the selected path.
Var f As FolderItem = FolderItem.ShowSaveFileDialog("","PDFMarkupExample.pdf")
If f <> Nil Then
d.Save(f)
End If
And this is how it looks when the resulting document is opened in Adobe Acrobat Reader:
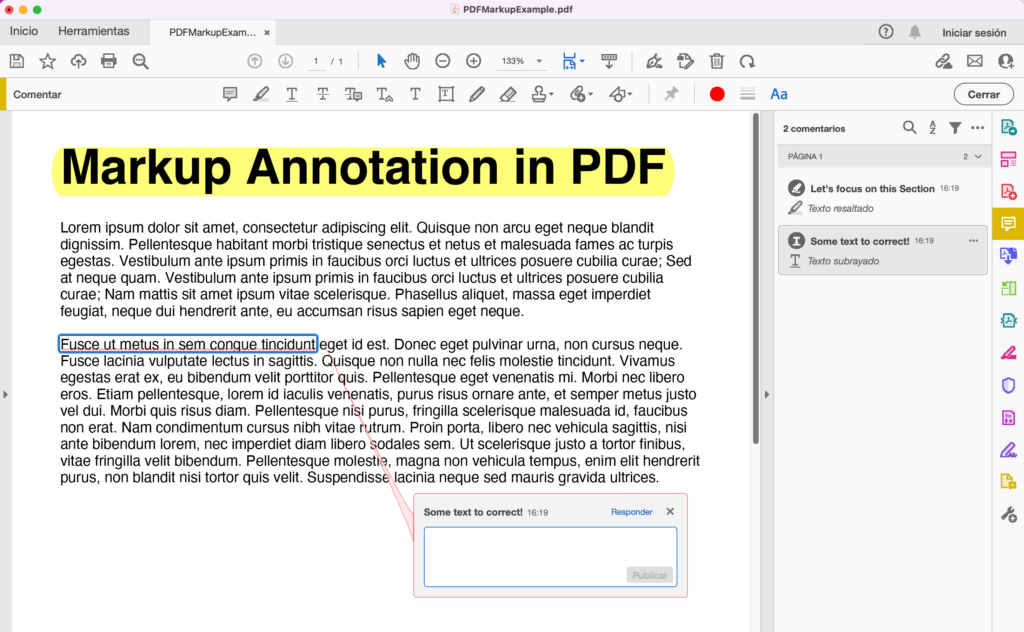
Bonus
Let me end with one other minor improvement to PDFDocument annotations: starting with Xojo 2022r2 all of them include metadata information about the creation and modification date and time. this information will be displayed as part of the annotation metadata when the item is selected in the viewer app.
Javier Menendez is an engineer at Xojo and has been using Xojo since 1998. He lives in Castellón, Spain and hosts regular Xojo hangouts en español. Ask Javier questions on Twitter at @XojoES or on the Xojo Forum.