In software development, efficiency and user experience are paramount. One powerful technique that can help achieve both is the use of type casting combined with iteration. By iterating through controls and casting them to specific types, developers can dynamically manipulate user interface elements, resulting in more responsive and interactive applications.
In this article, we will explore the concepts of type casting and iteration within Xojo applications. We will break down a practical example to demonstrate how these techniques can be used to optimize form handling and enhance user interactions.
Understanding Type Casting
Type casting is a concept where a generic control is converted—or “cast”—to a specific type to access its unique properties and methods. This technique is particularly useful when you need to manipulate various controls dynamically within a loop.
For instance, imagine you have multiple text fields on a Window and you want to apply specific actions only to some controls. By using type casting you can loop through all controls, identify those that you’re looking for, and then apply the desired actions.
Type casting is advantageous because it allows you to:
- Access Specific Properties: Gain access to properties and methods specific to the control type
- Enhance Code Maintainability: Write cleaner, more readable and reusable code
- Improve Performance: Perform operations more efficiently by targeting specific control types directly
In essence, type casting allows developers to create more interactive and user-friendly applications by leveraging the full capabilities of each control type (you will see later in our example).
Iterating Through UI Controls
Iteration is a fundamental programming technique used to repeat a block of code multiple times. In Xojo, iterating through controls allows developers to dynamically interact with each instanced control, making it possible to apply specific actions based on the control type.
To iterate through controls in Xojo, you can use a simple For Each loop, which simplifies the process of accessing each control individually. Here’s a basic example:
For Each control As DesktopUIControl In Self.Controls
// Perform actions on each control
Next
Now, let’s utilize type casting to identify and manipulate all DesktopUIControl type controls. For example, if you want to find all DesktopTextField controls and perform certain actions, like disabling them, you can do so as follows:
// Loop through all controls in the current window or container
For Each control As DesktopUIControl In Self.Controls
// Check if the current control is a DesktopTextField
If control IsA DesktopTextField Then
// Cast the control to a DesktopTextField for specific operations
Var dtfield As DesktopTextField = DesktopTextField(control)
// Disable the DesktopTextField type controls
dtfield.Enabled = False
End If
Next
Here’s a breakdown of what each part of the code does:
- The For Each loop iterates through all controls in Self.Controls. Self refers to the current window or container.
- The If statement checks if the current control is an instance of DesktopTextField.
- If it is a DesktopTextField type, the control is cast to a DesktopTextField variable named dtfield and then disable it.
Tip: Make sure to read more about the operator IsA at https://documentation.xojo.com/api/language/isa.html
Practical Example
Let’s get into a practical example to see type casting and iteration in action.
- Place different type controls on a Window (buttons, text fields, text areas, popup menus, etc.).
- Make sure to also place two DesktopTextField controls and name them “PasswordField” and “EmailField”.
- In a Button control, add the Pressed event and write the following code:
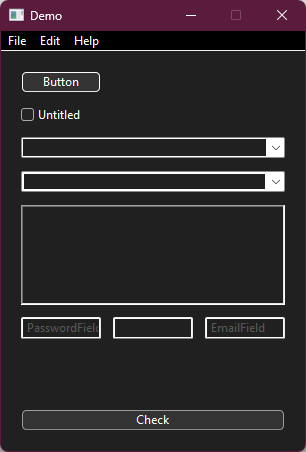
// Loop through all controls in the current Window or Container
For Each control As DesktopUIControl In Self.Controls
// Test if the current control is a DesktopTextField
If control IsA DesktopTextField Then
// Cast the control to a DesktopTextField for specific operations
Var dtfield As DesktopTextField = DesktopTextField(control)
// Get the name of the current text field
Var ctrlName As String = dtfield.Name
// Check if the text field is either the password field or email field
If (ctrlName = "PasswordField") Or (ctrlName = "EmailField") Then
// Check if the text field is empty
If dtfield.Text.IsEmpty = True Then
// If empty, set focus to this field
dtfield.SetFocus
// Exit the loop and the current method/function
Return
End If
End If
End If
Next
Code breakdown:
- The code starts with a For Each loop that iterates through all controls available in the Window/Container (Self.Controls).
- It checks if each control is a DesktopTextField.
- If it is, the control is cast to a DesktopTextField variable named dtfield.
- The name of the text field is stored in the ctrlName variable.
- The code then checks if the text field is either the “PasswordField” or “EmailField”.
- If it is one of these fields, it checks if the field is empty.
- If the field is empty, it sets the focus to this field and exits the current method or function using Return.
The above Xojo code snippet is used to perform a simple form validation. It checks if the password and email fields are filled out, and if either is empty, it sets the focus to the empty field and stops further processing. This is useful for ensuring that users don’t submit a form with these crucial fields left blank.
Conclusion
Mastering software development type casting and iteration not only makes your code more maintainable but it’s also a good strategy for dynamically managing instanced objects.
We encourage you to experiment with these techniques in your own Xojo projects. By applying the principles discussed in this blog post, you’ll be well on your way to creating more efficient and interactive applications.
Make sure to also check the following resources:
1. https://documentation.xojo.com/getting_started/object-oriented_programming/advanced_oop_features.html#casting
2. https://documentation.xojo.com/api/language/isa.html#isa
3. https://blog.xojo.com/2017/12/13/make-your-own-classes-iterables/
4. https://blog.xojo.com/2018/03/21/casting-get-ready-and-keep-the-type/
Gabriel is a digital marketing enthusiast who loves coding with Xojo to create cool software tools for any platform. He is always eager to learn and share new ideas!