On the forum a user asked if there was a way to create a rectangle with only the top left and top right corners being rounded. Xojo’s built-in RoundRectangle control draws with all four corners rounded, so that was not an option.
One solution is to use a GraphicsPath to draw exactly what you want. With a GraphicsPath you can use the AddArc() method to add rounded corners and then draw the lines for the rest of the rectangle.
This is the code for desktop:
Const Pi = 3.14159
Const x = 50
Const y = 00
Const rectHeight = 100
Const rectWidth = 100
Const arcRadius = 20
Var topRoundRect As New GraphicsPath
topRoundRect.AddArc(x, y, arcRadius, Pi, 1.5 * Pi, False)
topRoundRect.AddArc(x + rectWidth, y, arcRadius, 1.5 * Pi, 0, False)
topRoundRect.AddLineToPoint(x + rectWidth + arcRadius, rectHeight)
topRoundRect.AddLineToPoint(x - arcRadius, rectHeight)
topRoundRect.AddLineToPoint(x - arcRadius, y)
g.DrawPath(topRoundRect)
You’ll want to tweak the numbers to fit your situation, but this should give you the idea.
With a couple minor tweaks1, the same code also works on iOS. Change GraphicsPath to iOSPath and change the AddLineToPoint() method calls to LineToPoint(). This is the result:
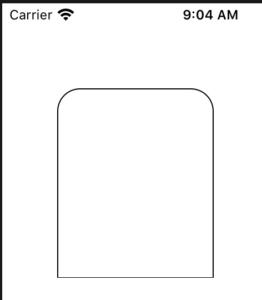
GraphicsPath maps very closely to HTML5 Canvas Path so you can often find code samples online that are easily adapted to Xojo. For example, take a look at this JavaScript code from HTML5 Canvas Tutorial which draws a squiggle:
var context = canvas.getContext('2d');
context.beginPath();
context.moveTo(100, 20);
// line 1
context.lineTo(200, 160);
// quadratic curve
context.quadraticCurveTo(230, 200, 250, 120);
// bezier curve
context.bezierCurveTo(290, -40, 300, 200, 400, 150);
// line 2
context.lineTo(500, 90);
context.lineWidth = 5;
context.strokeStyle = 'blue';
context.stroke();
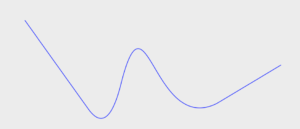
Notice how similar the JavaScript looks to the equivalent Xojo code that draws the same squiggle:
Var path As New GraphicsPath
path.MoveToPoint(100, 20)
// line 1
path.AddLineToPoint(200, 160)
// quadratic curve
path.AddQuadraticCurveToPoint(230, 200, 250, 120)
// bezier curve
path.AddCurveToPoint(290, -40, 300, 200, 400, 150)
// line 2
path.AddLineToPoint(500, 90)
g.DrawingColor = Color.Blue
g.DrawPath(path)
1 As we mentioned in the Xojo 2020 Update video and in our Roadmap, the iOS API is planned to match the API used by other platforms so you won’t need slightly different code here in the future.