I know, I know, it’s not the usual thing to do when making presentations, but did you know that it is possible to create presentations using Xojo’s and PDF? If you’re interested in this little experiment, read on and I’ll show you how to do it using Xojo’s PDFDocument.
Why, you ask? What are the advantages of using a PDF for your presentations? PDF is portable, meaning you can distribute these files easily, usually, with a small file footprint. The content is vectorial (except for images), what means that it will look great no matter the screen resolution of the display/projector used for playing the presentation.
There is one current issue to watch out for, currently there’s a bug on macOS where Acrobat Reader is not able to apply the assigned transitions on Big Sur and later; they still work under Catalina and previous versions of macOS.
In this example project I will create a shortened version of the slides I used in the Using Xojo’s PDFDocument presentation. You’ll need to change the font names (and any other item) to those you are using and that are installed in your computer.
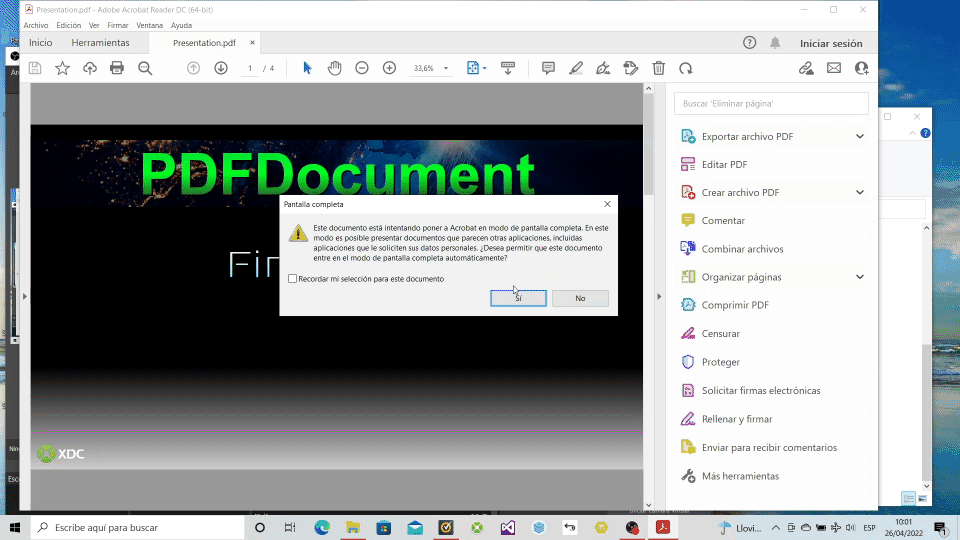
Setting the Viewer Options
Start by creating a new project for the target you’re most comfortable with – Xojo’s PDFDocument is cross-platform and supported on Desktop, Web, iOS and Console projects. Next, add the Opening Event Handler to the default DesktopWindow/WebWindow/MobileScreen, or choose the Run Event Handler if you chose to create a new Console Project.
Write the following lines of code in the associated Code Editor:
// Let's create a new PDFDocument instance with // the page size set to HD resolution Var Presentation As New PDFDocument(1920, 1080) // Let's make sure that the fonts used are embedded in the // document, so there are no unexpected problems when the // PDF is opened in a device that doesn't have that // same fonts installed Presentation.EmbeddedFonts = True
The next lines of code will set the default for the View mode of the document when it is opened in Acrobat Reader or any other PDF reader. Since it’s a presentation, you’ll likely want the document to be opened in Full Screen mode by default, so let’s make that happen:
// Let's create a new Instance from the PDFViewerOptions class Var ViewerOptions As New PDFViewerOptions // And set the FullScreen property to True // so the document will set that view size // when opened in the PDF Viewer app ViewerOptions.FullScreen = True
Drawing the Background of the Page
Use a linear gradient brush to fill in the background of all the slides. For convenience, create a new method for it using the following values in the Inspector Panel:
- Name: DrawPageBackground
- Parameters: g As Graphics
Type the following code in the associated Code Editor for the method:
Static backgroundfilling As LinearGradientBrush If backgroundfilling = Nil Then backgroundfilling = New LinearGradientBrush backgroundfilling.StartPoint = New Point(g.Width / 2, 0) backgroundfilling.EndPoint = New Point(g.Width / 2, g.Height) backgroundfilling.GradientStops.Add(New Pair(0.0, &c000000)) backgroundfilling.GradientStops.Add(New Pair(0.7, &c000000)) backgroundfilling.GradientStops.Add(New Pair(1.0, &ccccccc)) End If g.Brush = backgroundfilling g.FillRectangle(0, 0, g.Width, g.Height)
All this code does is create a LinearGradientBrush instance ranging from full black to some degree of gray at the bottom of the page. Because we are not going to change the values of the gradient every time we need to call the method, it makes sense to assign it to a Static instead of a Variable.
Then the LinearGradientBrush is assigned to the Brush property of the received Graphic context and, finally, used as the “filling color” when drawing the filled rectangle.
Drawing the Header
Now add the method that will be responsible for drawing the header shared by all the slides. Use the following values in the Inspector Panel:
- Method Name: DrawTopHeader
- Parameters: g As Graphics
And type the following code in the associated Code Editor:
Const kPDFDocumentHeader As String = "PDFDocument" DrawUpperBand(g, 0, 50) g.FontName = "Helvetica" g.FontSize = 180 g.Bold = True Var x As Double = g.Width / 2 - g.TextWidth(kPDFDocumentHeader) / 2 Var y As Double = 220 g.DrawingColor = &cC9F2C900 g.DrawText(kPDFDocumentHeader, x + 4, 224) g.DrawingColor = Color.Black Static linearGradient As LinearGradientBrush If linearGradient = Nil Then Lineargradient = New LinearGradientBrush linearGradient.StartPoint = New point(x, y - 224) linearGradient.EndPoint = New point(x, y) linearGradient.GradientStops.Add(New Pair(0.0, &c00BD4E00)) linearGradient.GradientStops.Add(New Pair(0.7, &c00FD1F00)) linearGradient.GradientStops.Add(New Pair(1.0, &c25712900)) End If g.Brush = LinearGradient g.DrawText(kPDFDocumentHeader, x, y)
As you can see, this is quite similar to the previous code. We are using a new Linear Gradient as the filling for the “PDFDocument” text. But first, it calls the DrawUpperBand method. This is a method that will draw a picture with a 50% opacity behind the header text, as well as the XDC logo in the lower left corner of the slide. Add a new method using the following values:
- Method Name: DrawUpperBand
- Parameters: g As Graphics, x As Integer, y As Integer
Type the following snippet of code in the associated Code Editor:
g.Transparency = 50.0 g.DrawPicture(UpperBand, x, y) g.Transparency = 0 g.DrawPicture(XDC2x, 20, g.Height - XDC2x.Height)
Both “UpperBand” and “XDC2x” are PNG image files added to the project.
NextPage
Because the background and the header are common items in all the slides, we will render them every time we add a new slide (page) to the presentation (document). Create a new method using the following values:
- Method Name: NextPage
- Parameters: g As Graphics
And type the following lines of code:
g.NextPage(1920, 1080) DrawPageBackground(g) DrawTopHeader(g)
Creating the Content of the Slides
Now let’s add the method that will be responsible for adding the text of the main topic on every slide:
- Method Name: DrawTopic
- Parameters: g As Graphics, topic As String
And type the following code in the associated Code Editor:
Var x, y As Double g.FontName = "Anoxic Light" g.FontSize = 150 x = g.Width / 2 - g.TextWidth(topic) / 2 y = g.Height / 2 - g.TextHeight / 2 Static LinearGradient As LinearGradientBrush If LinearGradient = Nil Then linearGradient = New LinearGradientBrush linearGradient.StartPoint = New Point(x, y) linearGradient.EndPoint = New Point(x, y - 80) linearGradient.GradientStops.Add(New pair(1.0, &c9FE1FF00)) linearGradient.GradientStops.Add(New pair(0.0, &cF6F6F600)) End If g.Brush = linearGradient g.DrawText(topic, x, y)
Setting Transitions Between Slides
PDFDocument does support the range of transitions available under the PDF standard. In this case we are going to use the simplest: Fade. So, create a new method with the following values:
- Method Name: AddTransitions
- Parameters: d as PDFDocument
And type the following lines of code in the associated Code Editor:
// New PDFTransition instance, using the Fade style // with one second of length. Var t As New PDFTransition(PDFTransition.Styles.Fade, 1) // And apply it on every page of the PDFDocument, starting on Page 2. If d.PageCount > 2 Then For n As Integer = 2 To d.PageCount d.TransitionAt(n) = t Next End If
Putting It All Together
Now we have all the pieces needed to create this small presentation in PDF. Go back to the Opening Event Handler and type the following lines of code below the last one:
DrawPageBackground(g) DrawTopHeader(g) DrawTopic(g, "First Slide") NextPage(g) DrawTopic(g, "Second Slide") NextPage(g) DrawTopic(g, "Third Slide") NextPage(g) DrawTopic(g, "Fourth Slide") AddTransitions(d) d.Save(SpecialFolder.Desktop.Child("Presentation.pdf"))
Done! Run the project and the PDF document will be saved to your computer Desktop. Once you open it in Adobe Reader, the app will detect that the document wants to use the full screen mode and, as soon as you confirm, it will begin the presentation. Depending on your preferences in Acrobat Reader, you may need to use the mouse button or arrow keys to change slides, or it may automatically advance to the next slide after the amount of time set.
Javier Menendez is an engineer at Xojo and has been using Xojo since 1998. He lives in Castellón, Spain and hosts regular Xojo hangouts en español. Ask Javier questions on Twitter at @XojoES or on the Xojo Forum.