Being able to call ChatGPT from inside your apps for specific purposes opens up a world of new possibilities. ChatGPT is an AI or more specifically a Large Language Model (LLM). To make using ChatGPT in Xojo easier, you can use the new ChatGPTConnection class. You can find an example project showing you how by launching Xojo, clicking on Examples in the Project Chooser and then looking in the AI section. Using this class, you can make use of ChatGPT from within your Xojo projects.
As an example of how you might use ChatGPT in Xojo, I looked for a way to turn statements into questions. So I ask ChatGPT:
Turn “numerical limits of double in xojo” into a sentence.
ChatGPT’s response is:
What are the numerical limits of the double data type in XOJO?
Getting Started
Before you can run the example to test out the ChatGPTConnection class or use it in your own projects, you’ll need to do three things:
- Create an account at OpenAI.com. This is free.
- Add a payment method to your account (to use ChatGPT via an API, you need pay – more on that in a bit).
- Get an API key. You’ll need to have added a payment method before you can get an API key.
One you have done this, open the ChatGPT Example project, open the ChatGPTConnection class and in the Inspector assign your API key to the APIKey constant. Now you can run the example. The example presents a window where you converse with ChatGPT.
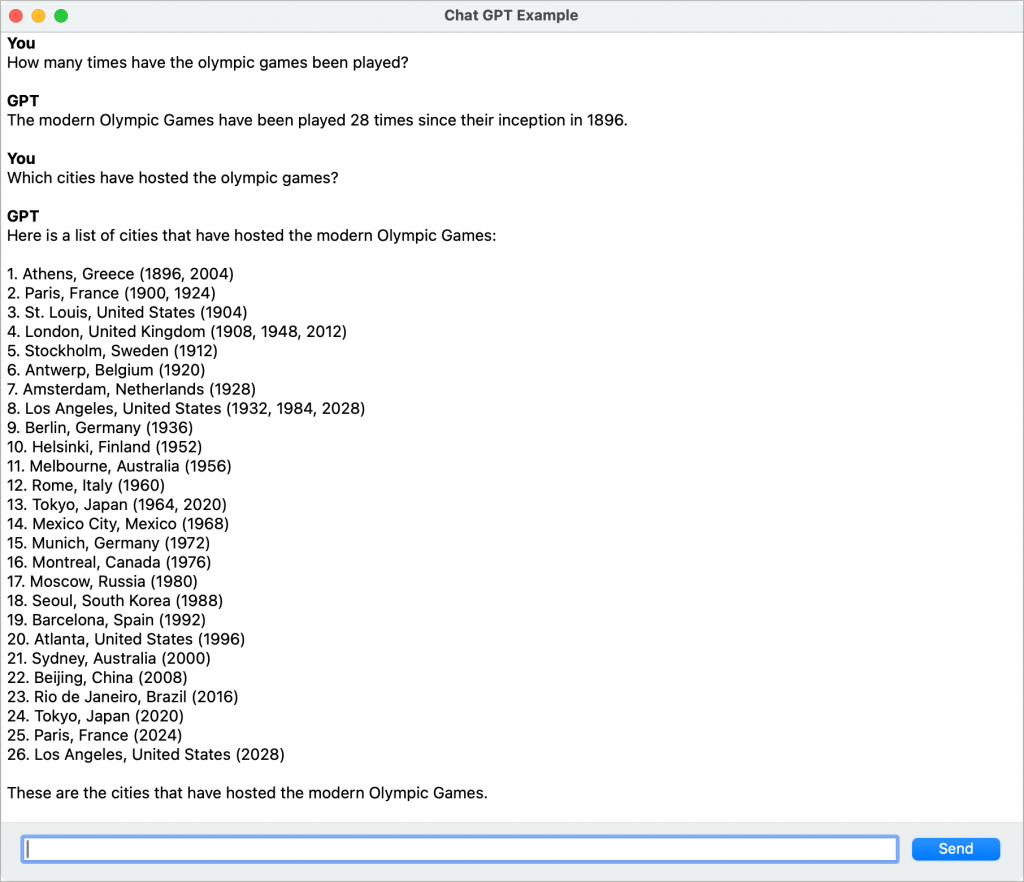
Each window is a separate context. Try choosing File > New Conversation. Referring to questions you asked in another window won’t work because in this example each has its own context.
Using the ChatGPT Classes in Your Project
To use the ChatGPTConnection class in your project, copy both the ChatGPTConnection and the ChatGPTException classes to your project.
To use the ChatGPTConnection class, either drag it onto a window, webpage or mobile screen or create an instance in code. Most of the work is done by a single method: ReplyToPrompt. The syntax for ReplyToPrompt is:
ReplyToPrompt(prompt As String, Optional MaintainContext As Boolean = False) As String
In its simplest form, just pass your prompt and the class will return ChatGPT’s response. For example:
Var gpt As New ChatGPTConnection
Var response As String = gpt.ReplyToPrompt("Where were the 1980 Olympic Games held?")
MessageBox(response)
When you want ChatGPT to consider past requests in the same session, pass True for the MaintainContext parameter. This tells the ChatGPTConnection class to keep a history of all your requests and the responses from ChatGPT which it then sends along with your new request each time you call the ReplyToPrompt method. The amount of text you can send is not unlimited so only set MaintainContext to True when you really do need it to consider previous requests and responses. ChatGPTConnection defaults to GPT 3.5 Turbo which has a maximum limit of 16,385 tokens per context. A token is a number of characters. The conversion is approximately 4 characters per token. Think of a token as a typical word. When you set the MaintainContext property to True, the ChatGPTConnection class monitors your usage of tokens. When it gets to 90% of the allowed maximum for the model you are using, it will start trimming the oldest requests and responses from your history (stored in the ContextHistory property) to avoid you going over the limit.
ChatGPTConnection Class Properties
You don’t need to set any of the properties in the ChatGPTConnection class as they all have useful default values. However, if you want to use a model different from the default (gpt-3.5-turbo), you can set the Model property before calling the ReplyToPrompt method. You can find a list of models here. Models have different limits as to how many tokens you can use so when you change the Model property, make sure to also change the MaximumTokensPerContext property to the appropriate value for the model. The Temperature property indicates how creative ChatGPT will be. Another way of saying this is how much it will hallucinate. Its value range is from 0 (don’t hallucinate at all) to 2 (hallucinate all you want). The default is 0.5. Finally, the TimeOut property is how long ReplyToPrompt will wait for a response. The default is 30 seconds.
If anything goes wrong, the ChatGPTConnection class will raise a ChatGPTException so make sure to put your calls to the ReplyToPrompt method in a Try Catch statement:
Try
Var gpt As New ChatGPTConnection
Var response As String = gpt.ReplyToPrompt("Where were the 1980 Olympic Games held?") MessageBox(response)
Catch e As ChatGPTException
MessageBox("Error: " + e.Message)
End Try
Pricing for ChatGPT is by millions of tokens. Chat GPT 3.5 is relatively inexpensive so if it gives you the results you want, use it. As of this writing, every 1 million words/tokens you send it will only cost 50 cents in US dollars. Every 1 million words/tokens you get back in response will cost $1.50 USD. If you need higher quality responses, consider Chat GPT 4.
Geoff Perlman is the Founder and CEO of Xojo. When he’s not leading the Xojo team he can be found playing drums in Austin, Texas and spending time with his family.