Back in 2015, I wrote a blog post about an iOS app called CatsUp! that used The Cat API to display random cat pictures. Things have changed a lot since then, so it’s time for an update!
With just a few lines of code, you can create a mobile app that shows a new cat picture each time you launch it. I call this app “CatsUp!”. It’s a play on ketchup/catsup, get it?
Set Up The Project
Create a new iOS project called “CatsUp”. Drag an ImageViewer control onto Screen1, make it fill most of the area and change its Name to “CatImage”. Drag a Button to the bottom and change its Caption to “Cat Me!”. Also change the Screen Title to be “CatsUp!”. The result should look something like this:
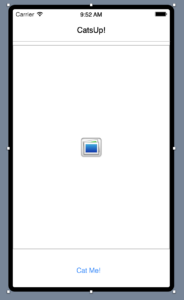
The Cat API
The Cat API documentation page describes the full API. For access to most features you will want to sign up for a free account and use an API key. For the purposes of this post, I will just use it without an API key. The URL for the method to get a random cat picture is:
https://api.thecatapi.com/v1/images/search
The above URL returns JSON that contains the location of a picture to display. Paste it into a web browser to try it. In my test I got this JSON:
[{"breeds":[],"id":"99v","url":"https://cdn2.thecatapi.com/images/99v.jpg","width":384,"height":384}]
To make CatsUp, we will essentially add some code to 1) send the first request, 2) get the JSON and pull out the picture URL, 3) get the picture and display it.
Add Code
Add the Pressed event to the Button with this code:
CatConnection.Send("GET", "https://api.thecatapi.com/v1/images/search")
This calls the Send() method on a URLConnection object, called CatConnection, to get the JSON with the picture URL.
So now you need to drag a URLConnection object from the Library onto the Screen. Click on this newly added URLConnection1 item in the Tray and in the Inspector change its name to “CatConnection”. Add its ContentReceived event with this code:
Var json As JSONItem = New JSONItem json.Load(content) Var catItem As JSONItem catItem = json.ValueAt(0) Var picURL As String picURL = catItem.Value("url").StringValue ImageDownload.Send("GET", picURL)
The above code loads the JSON into a JSONItem and then fetches the “url” value. It sends this URL to another URLConnection, called ImageDownload, to get the actual picture to display.
Drag a second URLConnection onto the screen and change its name to “ImageDownload”. Add its ContentReceived event with this code:
Var pic As Picture = Picture.FromData(content) CatImage.Image = pic
Try running the project in the iOS Simulator. Click the button to see a new cat picture.
This is what I see:
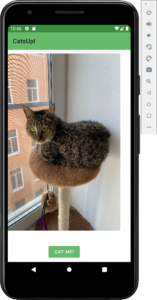
Oops, silly me. I actually created this as an Android project and ran it in the Android Emulator. Sorry about that, here is what it looks like as an iOS project running in the iOS Simulator:
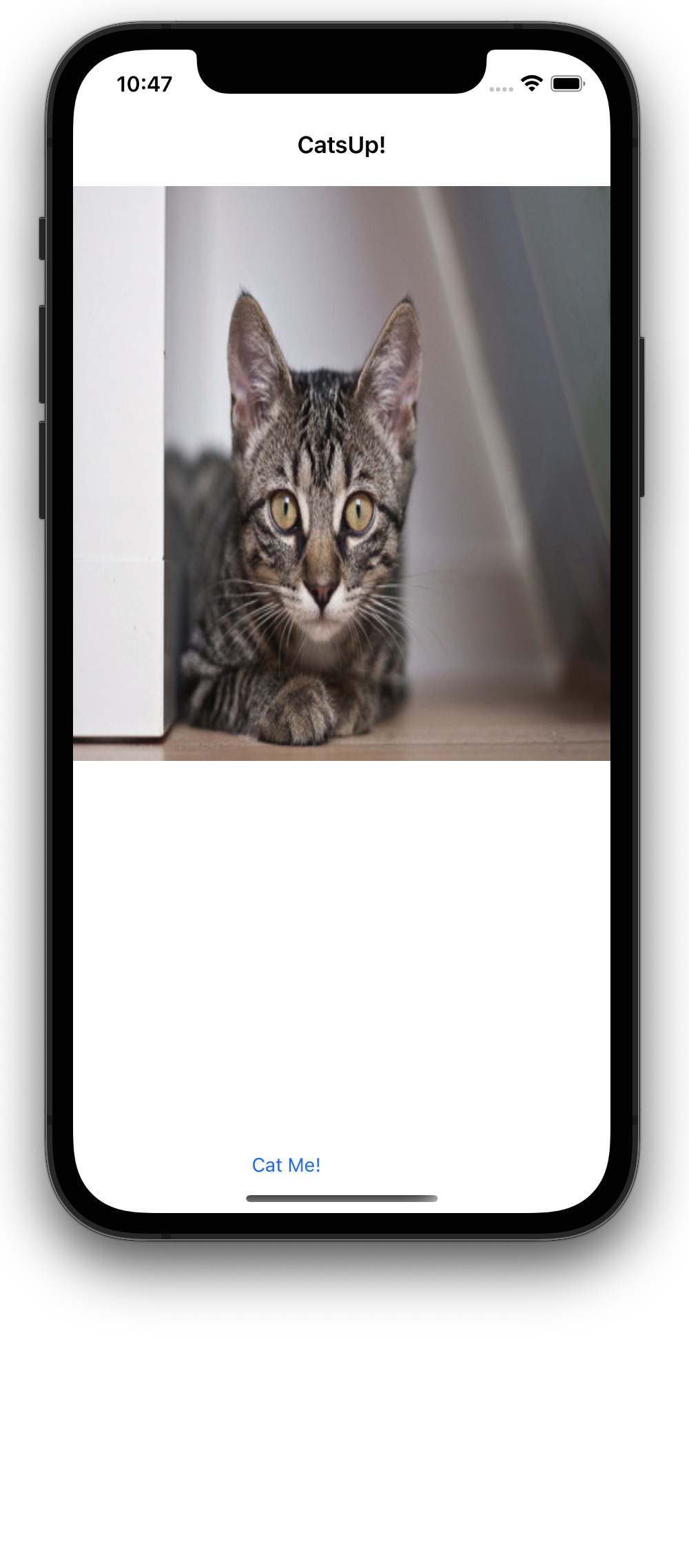
That’s right, the exact same code for this project can be used in both iOS and Android projects! And for another bit of Android news, this CatsUp! app, with a couple minor enhancements, is now available in the Google Play Store:
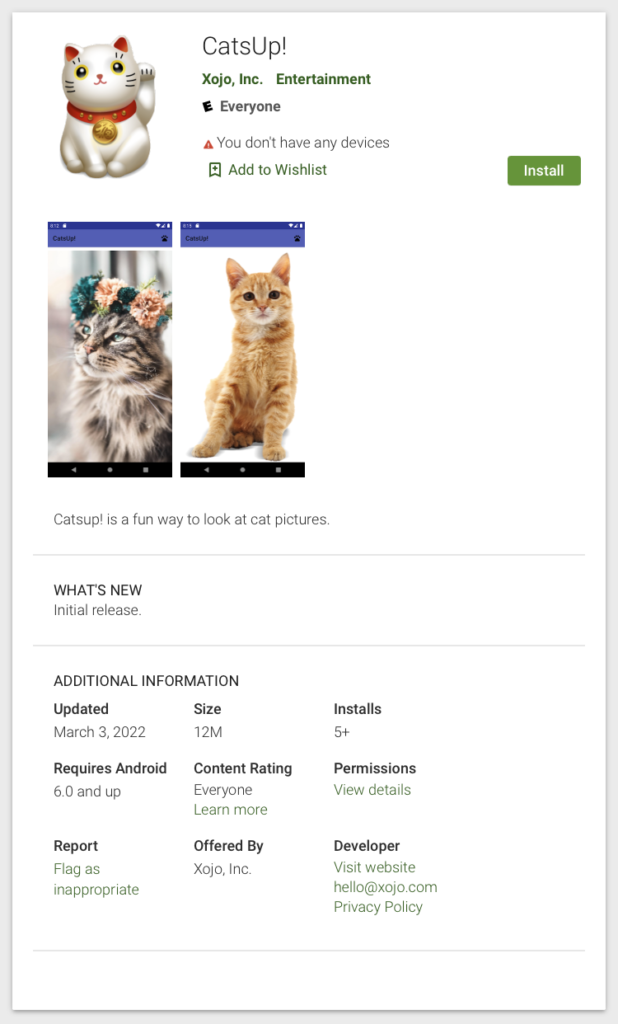
Have fun with this and may every day be a Caturday!